猫狗识别pytorch
时间: 2023-08-08 17:13:43 浏览: 45
对于猫狗识别的任务,你可以使用PyTorch来构建一个深度学习模型。下面是一个简单的示例代码:
首先,你需要导入所需的库:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision.datasets import ImageFolder
from torchvision.transforms import ToTensor
from torch.utils.data import DataLoader
```
然后,定义一个自定义的卷积神经网络模型:
```python
class CatDogClassifier(nn.Module):
def __init__(self):
super(CatDogClassifier, self).__init__()
self.conv1 = nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(64 * 56 * 56, 128)
self.fc2 = nn.Linear(128, 2)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = nn.functional.max_pool2d(x, 2)
x = nn.functional.relu(self.conv2(x))
x = nn.functional.max_pool2d(x, 2)
x = nn.functional.relu(self.conv3(x))
x = nn.functional.max_pool2d(x, 2)
x = x.view(x.size(0), -1)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
```
接下来,加载数据集并进行数据预处理:
```python
train_dataset = ImageFolder('path/to/train/dataset', transform=ToTensor())
test_dataset = ImageFolder('path/to/test/dataset', transform=ToTensor())
train_loader = DataLoader(train_dataset, batch_size=16, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=16, shuffle=False)
```
然后,定义模型、损失函数和优化器:
```python
model = CatDogClassifier()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
接下来,进行模型训练:
```python
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model.to(device)
num_epochs = 10
for epoch in range(num_epochs):
model.train()
for images, labels in train_loader:
images, labels = images.to(device), labels.to(device)
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
model.eval()
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
images, labels = images.to(device), labels.to(device)
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f'Epoch {epoch+1}, Test Accuracy: {accuracy:.2f}%')
```
最后,你可以使用训练好的模型进行预测:
```python
def predict(image_path):
model.eval()
with torch.no_grad():
image = Image.open(image_path)
image = ToTensor()(image).unsqueeze(0)
image = image.to(device)
output = model(image)
_, predicted = torch.max(output.data, 1)
return predicted.item()
```
这是一个简单的猫狗识别任务的PyTorch示例代码,你可以根据实际需求进行调整和扩展。
相关推荐
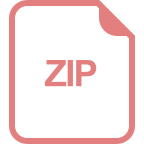














