帮我写一个用折半查找法找十五个数字中数字在数字中位置的程序
时间: 2024-12-15 14:20:28 浏览: 11
折半查找(Binary Search),也称为二分查找,适用于已经排序的数据列表。在这个例子中,我们需要先假设给定的15个数字已经按升序排列。下面是使用C语言编写的一个简单的折半查找函数,用于找到特定数字的位置:
```c
#include <stdio.h>
// 假设数组名为arr,大小为15,元素已排序
int binary_search(int arr[], int target, int low, int high) {
if (low <= high) {
int mid = low + (high - low) / 2;
// 检查中间元素是否等于目标
if (arr[mid] == target)
return mid; // 找到位置并返回
// 如果目标小于中间元素,则在左半边继续搜索
else if (arr[mid] > target)
return binary_search(arr, target, low, mid - 1);
// 否则,在右半边继续搜索
else
return binary_search(arr, target, mid + 1, high);
}
// 目标不在数组中,返回-1表示不存在
return -1;
}
int main() {
int arr[15] = {1, 3, 4, 6, 7, 9, 10, 12, 13, 15, 17, 18, 19, 21, 23};
int target = 10; // 我们要查找这个数字在数组中的位置
int position = binary_search(arr, target, 0, 14); // 注意数组下标从0开始
if (position != -1)
printf("Number %d is at index %d\n", target, position + 1);
else
printf("Number %d not found in the array.\n", target);
return 0;
}
```
阅读全文
相关推荐


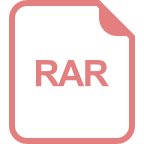















