集训队在判题的时候,经常发现选手把"Yes"输出成"YES"或者"yes",她希望写一个spj(special judge)来判断选手是否输出了"Yes"。你能帮帮她吗?c语言
时间: 2024-12-19 11:16:36 浏览: 11
当然可以。在C语言中,你可以编写一个简单的字符串比较函数来帮助判题。这里是一个基本的例子,它会检查输入的字符串是否只包含大写的 "Y" 和小写的 "e",并且它们之间只有一个空格:
```c
#include <stdbool.h>
#include <stdio.h>
#include <string.h>
bool isYes(const char *input) {
// 检查字符串长度是否为4,因为 "Yes" 有三个字符加上结尾的空字符 '\0'
if (strlen(input) != 4) {
return false;
}
// 确保首字母为 'Y',第二个字母为 'e',第三个字母也为 's'
if (input[0] != 'Y' || input[1] != 'e' || input[2] != 's') {
return false;
}
// 检查中间是否有空格
if (input[1] != ' ' || input[3] != '\0') {
return false;
}
return true;
}
int main() {
const char* test_cases[] = {"Yes", "yes", "yEs", "yeS", "YES", "y es"};
int num_test_cases = sizeof(test_cases) / sizeof(test_cases[0]);
for (int i = 0; i < num_test_cases; i++) {
bool result = isYes(test_cases[i]);
if (result) {
printf("Test case %d: Yes\n", i + 1);
} else {
printf("Test case %d: No\n", i + 1);
}
}
return 0;
}
```
这个 `isYes` 函数用于判断给定的字符串是否符合 "Yes" 的标准格式。如果满足条件,返回 `true`,否则返回 `false`。
阅读全文
相关推荐
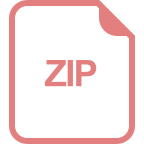
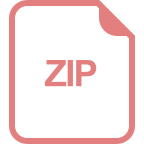

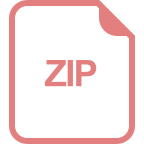
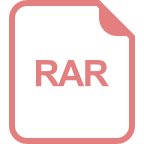









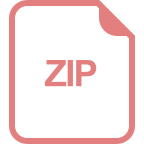
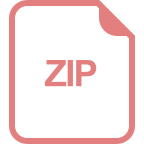