C++中有没有其他方法将浮点数字符串转换?
时间: 2024-11-20 09:36:23 浏览: 24
在C++中,确实有将浮点数字符串转换的方法,你可以使用`std::stod`(string to double)函数将其转换为双精度浮点数(double),如果需要更大范围的数值,可以使用`std::stof`(string to float)或者`std::stold`(string to long double)。以下是基本的用法示例:
```cpp
#include <iostream>
#include <string>
main() {
std::string strFloat = "3.14"; // 浮点数字符串
double doubleValue = std::stod(strFloat);
if (std::cin >> strFloat) { // 从输入获取
float floatValue = std::stof(strFloat);
long double lDoubleValue = std::stold(strFloat);
}
return 0;
}
```
同样,如果字符串不能解析为有效的浮点数,这些函数也会抛出异常。
相关问题
C++中有没有其他方式可以控制浮点数的输出精度?
除了使用`std::fixed`和`std::setprecision`组合之外,C++标准库并没有提供直接针对单个浮点数变量设置输出精度的方法。但是你可以选择使用`std::stringstream`或者第三方库,如`boost`的`lexical_cast`等来控制浮点数的精度。
例如,使用`std::stringstream`,你可以先转换成字符串,然后手动指定宽度和精度:
```cpp
#include <sstream>
#include <iomanip>
double value = 3.14159265358979;
std::ostringstream oss;
oss << std::setfill('0') << std::fixed << std::setw(10) << std::setprecision(6) << value;
std::string formatted_value = oss.str();
```
这里设置了输出值总共有10位,其中有6位小数。
另外,Boost库提供了`boost::format`或者`boost::lexical_cast<std::string>`等工具也能实现类似的功能。
c++将字符串转换为浮点数类型
可以使用C++中的stof函数将字符串转换为浮点数类型。示例如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float f = std::stof(str);
std::cout << "float value: " << f << std::endl;
return 0;
}
```
输出:
```
float value: 3.14
```
除了stof函数,还有stod和stold函数可以分别将字符串转换为双精度浮点数和长双精度浮点数。
阅读全文
相关推荐



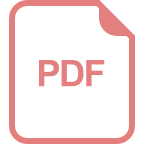












