用c++实现对双向链表的增删改查,数据包括,姓名,学号,并要求输出所有数据
时间: 2024-09-23 12:14:45 浏览: 37
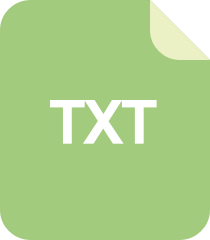
c++实现链表增删改查

在C++中,我们可以使用结构体(struct)来表示学生的姓名和学号,并创建一个双向链表(Doubly Linked List)来存储学生信息。这里是一个简单的示例,展示了如何实现链表的基本操作:
```cpp
#include <iostream>
using namespace std;
// 定义学生信息结构体
struct Student {
string name;
int id;
struct Node* prev; // 指向前一个节点
struct Node* next; // 指向下一个节点
};
// 双向链表节点结构体
struct Node {
Student data;
Node* prev;
Node* next;
};
// 初始化链表为空
Node* createEmptyList() {
return nullptr;
}
// 插入新节点到链表尾部
void insert(Student newStudent, Node*& head) {
Node* newNode = new Node();
newNode->data = newStudent;
newNode->prev = nullptr;
newNode->next = head;
if (head != nullptr) {
head->prev = newNode;
}
head = newNode;
}
// 删除指定学生的信息
void deleteStudent(int id, Node*& head) {
Node* current = head;
while (current != nullptr && current->data.id != id) {
current = current->next;
}
if (current == nullptr) {
cout << "Student not found." << endl;
return;
}
if (current->prev != nullptr) {
current->prev->next = current->next;
} else {
head = current->next;
}
if (current->next != nullptr) {
current->next->prev = current->prev;
}
delete current;
}
// 查找并打印指定学号的学生信息
void searchAndPrint(int id, const Node* head) {
Node* current = head;
while (current != nullptr) {
if (current->data.id == id) {
cout << "Name: " << current->data.name << ", ID: " << current->data.id << endl;
break;
}
current = current->next;
}
}
// 打印链表的所有学生信息
void printAll(Node* head) {
Node* current = head;
while (current != nullptr) {
cout << "Name: " << current->data.name << ", ID: " << current->data.id << endl;
current = current->next;
}
}
int main() {
Node* head = createEmptyList();
// 添加一些学生
insert({"Alice", 1001}, head);
insert({"Bob", 1002}, head);
insert({"Charlie", 1003}, head);
// 打印所有学生
printAll(head);
// 删除ID为1002的学生
deleteStudent(1002, head);
// 再次打印所有学生
printAll(head);
return 0;
}
```
这个程序实现了双向链表的插入、删除、查找和遍历功能。注意这只是一个基础示例,实际应用中可能需要处理更多的边界情况和错误检查。
阅读全文
相关推荐
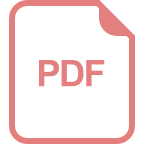
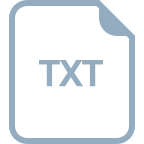

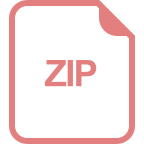
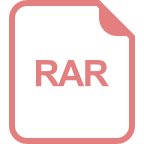
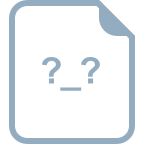
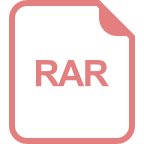
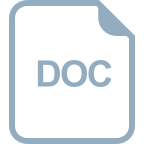
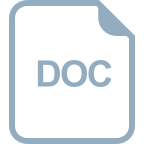
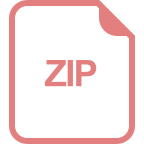
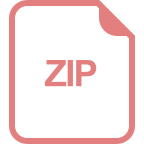
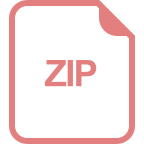
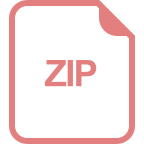
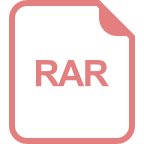