装箱算法 python
时间: 2023-08-19 07:04:45 浏览: 56
装箱算法是一种用来解决装箱问题的算法。其中一种叫做Bottom-Left装箱算法,它的思想是从箱子的右上角开始,先尽可能向下移动,再向左移动,不断循环直到无法再移动。这个算法可以用来优化箱子的空间利用率。在Python中,可以使用以下代码来实现Bottom-Left装箱算法:
```python
from tools import *
import random
#***(bottom-up left-justified)法求解二维装箱问题
# @BetterBench
# 思想:首先将选中的物体放在箱子的右上角,然后尽量向下向左作连续移动,直到不能移动为止
# 输入参数
itemNum = 30 # 物品数目
AllItem = np.array([[
相关问题
bottom-left装箱算法 python
Bottom-left装箱算法是一种常用的装箱算法,它主要用于解决物品装箱问题。该算法的思想是,将物品按照从大到小的次序依次放入一个矩形容器,每次放入后,将物品放在当前已经放置物品的最左下角的位置。
在Python中实现该算法可以按照以下步骤进行:
1. 首先定义一个矩形容器的初始大小,可以使用二维列表或者类来表示。例如,可以使用一个列表来表示容器的二维坐标系统,每个位置用0表示为空,1表示已经放置了物品。
2. 接下来,需要定义一个函数来判断是否能够将一个物品放入容器中。可以使用遍历的方式,从容器的左下角开始,逐行逐列检查容器中是否有足够的空间来放置该物品。如果有足够的空间,则可以将物品放入容器中,并将容器中相应位置的标记值设为1。
3. 在放置物品时,可以按照从大到小的次序依次放入。可以使用一个列表或者元组来存储物品的大小信息,并对其进行排序。
4. 最后,可以通过调用函数来模拟物品的放置过程,输出能够放入容器中的物品的摆放位置。
需要注意的是,bottom-left装箱算法是一种启发式算法,不能保证得到最优解。因此,在实际应用中,可能需要根据具体情况选择适合的算法来解决装箱问题。
装箱问题 算法 python
装箱问题是一个经典的组合优化问题,目标是将一组物品放入尽可能少的箱子中。这个问题在物流和运输领域中非常常见,可以使用一些启发式算法来解决。
一种常见的启发式算法是首次适应算法(First Fit Algorithm)。它按顺序将物品放入第一个能容纳下该物品的箱子中。如果没有箱子能容纳该物品,则打开一个新的箱子并将物品放入其中。
下面是一个使用 Python 实现的装箱算法示例:
```python
def first_fit(items, bin_capacity):
bins = []
for item in items:
# 尝试将物品放入已有的箱子中
for bin in bins:
if bin + item <= bin_capacity:
bin.append(item)
break
else:
# 如果无法放入任何已有箱子中,则打开新箱子
bins.append([item])
return bins
# 测试示例
items = [4, 8, 1, 2, 5, 3]
bin_capacity = 10
packed_bins = first_fit(items, bin_capacity)
print(f"需要的箱子数量:{len(packed_bins)}")
for i, bin in enumerate(packed_bins):
print(f"箱子 {i+1} 中的物品:{bin}")
```
此示例中,我们使用首次适应算法将物品 `[4, 8, 1, 2, 5, 3]` 放入容量为 10 的箱子中。输出结果将显示需要的箱子数量以及每个箱子中的物品。
请注意,这只是一种启发式算法,可能不一定能得到最优解。如果需要更精确的解决方案,可以尝试使用其他算法,如禁忌搜索、遗传算法等。
相关推荐
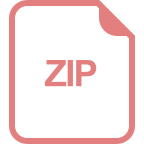
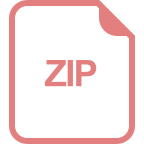
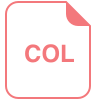
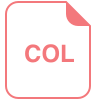
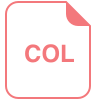
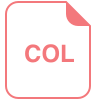
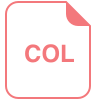







