FIFO算法LRU算法实验c语言
时间: 2023-11-28 08:45:29 浏览: 87
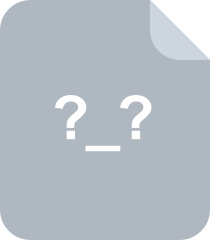
基于C语言的FIFO和LRU算法
下面是FIFO算法和LRU算法的C语言实现:
FIFO算法实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 3
int queue[MAXSIZE];
int front = 0, rear = 0;
void enqueue(int data) {
if ((rear + 1) % MAXSIZE == front) {
printf("队列已满,无法插入!\n");
return;
}
queue[rear] = data;
rear = (rear + 1) % MAXSIZE;
}
int dequeue() {
if (front == rear) {
printf("队列为空,无法删除!\n");
return -1;
}
int data = queue[front];
front = (front + 1) % MAXSIZE;
return data;
}
int main() {
enqueue(1);
enqueue(2);
enqueue(3);
printf("队列中的元素为:");
while (front != rear) {
printf("%d ", dequeue());
}
printf("\n");
return 0;
}
```
LRU算法实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 3
typedef struct {
int key;
int value;
} Node;
Node cache[MAXSIZE];
int count = 0;
void add(int key, int value) {
if (count == MAXSIZE) {
printf("缓存已满,需要进行替换!\n");
int min_index = 0;
for (int i = 1; i < MAXSIZE; i++) {
if (cache[i].key < cache[min_index].key) {
min_index = i;
}
}
cache[min_index].key = key;
cache[min_index].value = value;
} else {
cache[count].key = key;
cache[count].value = value;
count++;
}
}
int get(int key) {
for (int i = 0; i < count; i++) {
if (cache[i].key == key) {
int value = cache[i].value;
for (int j = i; j > 0; j--) {
cache[j].key = cache[j - 1].key;
cache[j].value = cache[j - 1].value;
}
cache[0].key = key;
cache[0].value = value;
return value;
}
}
printf("缓存中不存在该元素!\n");
return -1;
}
int main() {
add(1, 100);
add(2, 200);
add(3, 300);
printf("缓存中的元素为:\n");
for (int i = 0; i < count; i++) {
printf("%d: %d\n", cache[i].key, cache[i].value);
}
printf("获取元素2的值为:%d\n", get(2));
printf("缓存中的元素为:\n");
for (int i = 0; i < count; i++) {
printf("%d: %d\n", cache[i].key, cache[i].value);
}
return 0;
}
```
阅读全文
相关推荐


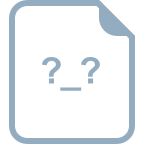
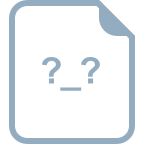
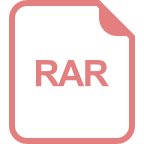
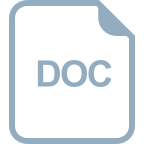
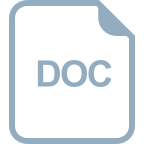
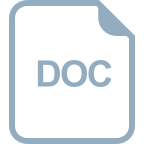
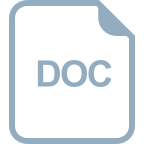
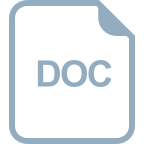





