#include <bits/stdc++.h> using namespace std; int main () { int n; string str; cin>>n;getchar(); while(n--){ getline(cin,str); cout<<str<<endl; str=regex_replace(str,regex(R"(\s+)")," "); str=regex_replace(str,regex(R"(^\s+|\s+$|\s+(?=\W))"),""); for(auto &c:str)if(c!='I')c=tolower(c); str=regex_replace(str,regex(R"(\bI\b)"),"&&&"); //将I me替换为&&&,避免影响can you和could you str=regex_replace(str,regex(R"(\bme\b)"),"&&&"); str=regex_replace(str,regex(R"(\bcan you\b)"),"I can"); str=regex_replace(str,regex(R"(\bcould you\b)"),"I could"); str=regex_replace(str,regex(R"(&&&)"),"you"); //&&& 转为 you str=regex_replace(str,regex(R"(\?)"),"!"); cout<<"AI: "<<str<<endl; } return 0; } 用C语言写
时间: 2023-07-17 21:08:21 浏览: 70
这段代码使用C++语言编写,而不是C语言。它是一个简单的程序,通过命令行输入一个整数n,然后循环n次,每次从输入中获取一行字符串。然后,它对字符串进行一系列的处理和替换操作,并输出处理后的结果。
具体的处理步骤如下:
- 使用getline函数获取一行输入字符串。
- 使用regex_replace函数和正则表达式将字符串中多个连续空格替换为单个空格。
- 使用regex_replace函数和正则表达式去除字符串两端的空格,以及在标点符号前后的空格。
- 将字符串中所有的大写字母'I'替换为小写字母'i'。
- 将字符串中独立的单词'I'和'me'替换为'&'字符,避免影响到'can you'和'could you'的处理。
- 将字符串中独立的单词'can you'替换为'I can'。
- 将字符串中独立的单词'could you'替换为'I could'。
- 将字符串中所有的'&'字符替换回'you'。
- 将字符串中所有的问号'?'替换为感叹号'!'。
- 输出处理后的字符串。
请注意,该代码依赖于C++标准库中的一些功能,包括iostream、string和regex等头文件。因此,在编译运行之前,请确保你的编译环境支持这些库。
相关问题
#include<bits/stdc++.h> #include<cstring> #include<sstream> using namespace std; bool cmp(string x,string y) { return atoi(x.c_str())>atoi(y.c_str()); } int main() { int n; cin>>n; string str=to_string(n); sort(str.begin(), str.end(), cmp); cout<<stoi(str); return 0; }
这段代码是一个简单的整数排序程序,它将输入的整数按照降序排列后输出。以下是对代码的解释:
1. 头文件部分:
```cpp
#include<bits/stdc++.h>
#include<cstring>
#include<sstream>
```
这部分包含了一些需要使用的标准库和头文件。
2. 比较函数:
```cpp
bool cmp(string x,string y)
{
return atoi(x.c_str())>atoi(y.c_str());
}
```
这是一个自定义的比较函数,用于在排序时判断两个字符串代表的整数的大小。它将字符串转换为整数后进行比较。
3. 主函数:
```cpp
int main()
{
int n;
cin>>n;
string str=to_string(n);
sort(str.begin(), str.end(), cmp);
cout<<stoi(str);
return 0;
}
```
在主函数中,首先从标准输入读取一个整数n。然后,使用`to_string`将整数n转换为字符串str。
接下来,使用`sort`函数对字符串str进行排序,排序时使用了自定义的比较函数cmp。
最后,使用`stoi`函数将排序后的字符串str转换为整数,并将结果输出到标准输出。
请注意,这段代码可能存在一些问题,例如没有对输入进行验证和错误处理。在实际使用中,应该进行适当的输入验证和错误处理来确保程序的健壮性。
请修改这段代码:#include<bits/stdc++.h> #include<cstring> #include<sstream> using namespace std; bool cmp(string x,string y) { return atoi(x.c_str())>atoi(y.c_str()); } int main() { int n; cin>>n; string str=to_string(n); sort(str.begin(), str.end(), cmp); cout<<stoi(str); return 0; }
以下是修改后的代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool cmp(char x, char y)
{
return x > y;
}
int main()
{
int n;
cin >> n;
string str = to_string(n);
vector<char> digits(str.begin(), str.end());
sort(digits.begin(), digits.end(), cmp);
string sortedStr(digits.begin(), digits.end());
int sortedNum = stoi(sortedStr);
cout << sortedNum;
return 0;
}
```
修改说明:
1. 移除了无关的头文件 `<bits/stdc++.h>` 和 `<cstring>`。
2. 添加了头文件 `<iostream>` 和 `<vector>`。
3. 修改了比较函数 `cmp`,将其参数由 `string` 类型改为 `char` 类型,以便在排序时直接对字符进行比较。
4. 使用 `vector<char>` 来保存整数的各个位上的数字。
5. 将排序后的字符数组转换为字符串 `sortedStr`。
6. 使用 `stoi` 将排序后的字符串转换为整数 `sortedNum`。
7. 输出排序后的整数。
请注意,这只是对原始代码的修改,不包含输入验证和错误处理。在实际使用中,应该添加适当的输入验证和错误处理来提高程序的健壮性。
相关推荐
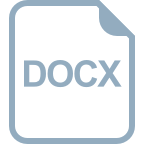
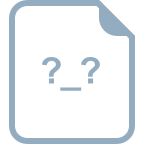
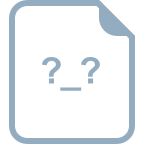













