设计模式+备忘录模式
时间: 2023-11-30 22:43:01 浏览: 110
备忘录模式是一种行为型设计模式,它允许在不破坏封装性的前提下捕获并保存一个对象的内部状态,并在需要时恢复该状态。在软件开发中,备忘录模式通常用于实现撤销操作或者历史记录功能。
下面是一个使用备忘录模式的简单示例,假设我们有一个文本编辑器,用户可以在其中输入文本并进行编辑操作。我们希望实现一个撤销功能,使得用户可以撤销之前的编辑操作。
首先,我们需要定义一个备忘录类,用于保存编辑器的状态:
```python
class EditorMemento:
def __init__(self, content):
self.content = content
def get_content(self):
return self.content
```
然后,我们需要定义一个编辑器类,其中包含了一些编辑操作,以及用于保存和恢复状态的方法:
```python
class Editor:
def __init__(self):
self.content = ""
def type(self, words):
self.content = self.content + " " + words
def delete(self, words):
if words in self.content:
self.content = self.content.replace(words, "")
def save(self):
return EditorMemento(self.content)
def restore(self, memento):
self.content = memento.get_content()
```
最后,我们可以使用这个编辑器类来实现撤销功能:
```python
editor = Editor()
editor.type("This is the first sentence.")
editor.type("This is the second sentence.")
saved = editor.save()
editor.delete("second")
print(editor.content) # 输出:This is the first sentence.
editor.restore(saved)
print(editor.content) # 输出:This is the first sentence. This is the second sentence.
```
阅读全文
相关推荐




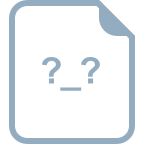











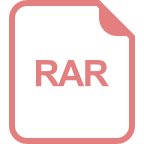
