请编写一段基于C++,Qt的VS程序,在子线程中调用opencv实现图片的阈值分割并显示
时间: 2024-04-15 16:29:29 浏览: 124
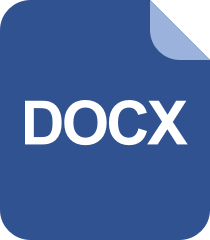
用vc实现图像阈值分割.docx
当然,下面是一段基于C++和Qt的VS程序代码,实现在子线程中调用OpenCV库实现图片的阈值分割并显示:
```cpp
#include <QApplication>
#include <QLabel>
#include <QThread>
#include <opencv2/opencv.hpp>
using namespace cv;
class ImageProcessingThread : public QThread
{
public:
explicit ImageProcessingThread(const QString& imagePath, QLabel* label)
: imagePath(imagePath), label(label)
{
}
void run() override
{
// 加载图像
Mat image = imread(imagePath.toStdString());
if (image.empty())
{
emit error("无法加载图像!");
return;
}
// 阈值分割
int thresholdValue = 128;
Mat thresholdedImage;
cvtColor(image, thresholdedImage, COLOR_BGR2GRAY);
threshold(thresholdedImage, thresholdedImage, thresholdValue, 255, THRESH_BINARY);
// 显示结果
QImage qImage(thresholdedImage.data, thresholdedImage.cols, thresholdedImage.rows, QImage::Format_Grayscale8);
QPixmap pixmap = QPixmap::fromImage(qImage);
emit imageProcessed(pixmap);
}
signals:
void imageProcessed(const QPixmap& pixmap);
void error(const QString& errorMessage);
private:
QString imagePath;
QLabel* label;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建一个窗口和一个标签用于显示图像
QWidget window;
QLabel label(&window);
label.setAlignment(Qt::AlignCenter);
window.resize(800, 600);
window.show();
// 创建图像处理线程
QString imagePath = "image.jpg";
ImageProcessingThread thread(imagePath, &label);
// 连接信号槽,当图像处理完成时更新标签中的图像
QObject::connect(&thread, &ImageProcessingThread::imageProcessed, [&label](const QPixmap& pixmap) {
label.setPixmap(pixmap.scaled(label.size(), Qt::KeepAspectRatio));
});
// 连接信号槽,当出现错误时显示错误消息
QObject::connect(&thread, &ImageProcessingThread::error, [](const QString& errorMessage) {
qDebug() << errorMessage;
});
// 启动图像处理线程
thread.start();
return a.exec();
}
```
这段代码基于Qt框架,使用了OpenCV库和Qt的多线程机制。在这个例子中,我们创建了一个窗口和一个标签用于显示图像,然后创建了一个继承自QThread的自定义线程类ImageProcessingThread。在ImageProcessingThread的run函数中,我们加载了指定路径下的图像,进行阈值分割并将结果以信号的形式发送出去。主线程中,我们将标签与图像处理线程的信号槽连接起来,当图像处理完成时更新标签中的图像。
请确保你已经安装了OpenCV库和Qt框架,并将图像文件"image.jpg"放置在正确的路径下,以便程序能够正确加载图像。
阅读全文
相关推荐
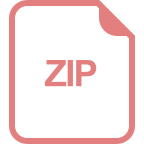
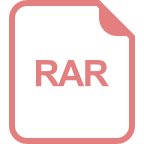
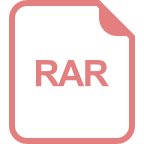
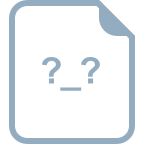
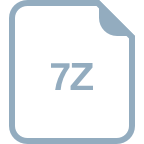
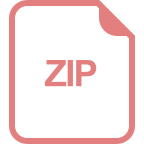
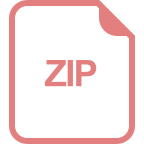
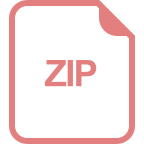
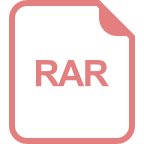
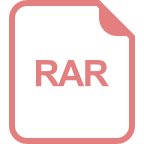
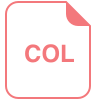
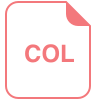
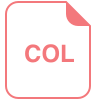
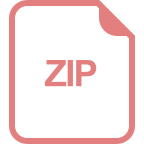