python实现将windows文件上传到windows
时间: 2024-10-18 18:13:55 浏览: 24
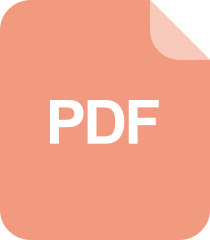
Python实现Windows和Linux之间互相传输文件(文件夹)的方法
在Python中,你可以使用内置的`os`模块配合`ftplib`库来实现Windows系统上文件的上传。如果你的目标是通过FTP协议上传文件,可以按照以下步骤操作:
1. 导入需要的库:
```python
import os
import ftplib
```
2. 配置FTP服务器连接信息,包括主机名、用户名、密码以及端口号:
```python
host = "your_ftp_host" # 例如:'192.168.1.1'
username = "your_username"
password = "your_password"
port = 21 # FTP默认端口
# 创建FTP客户端
ftp = ftplib.FTP()
ftp.connect(host, port)
ftp.login(username, password)
```
3. 切换到目标目录(如果需要):
```python
target_directory = "/path/to/destination/directory" # 替换为实际的路径
ftp.cwd(target_directory)
```
4. 读取本地文件并上传:
```python
local_file_path = "C:/path/to/local/file.txt" # 替换为你想要上传的文件路径
with open(local_file_path, 'rb') as file:
filename = os.path.basename(local_file_path) # 获取文件名
ftp.storbinary(f'STOR {filename}', file)
```
5. 关闭FTP连接:
```python
ftp.quit()
```
完成以上步骤后,Python程序就实现了从Windows本地文件系统的文件上传到远程Windows FTP服务器。
阅读全文
相关推荐
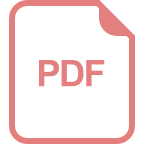
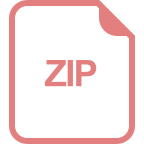
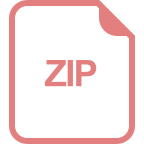
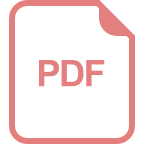
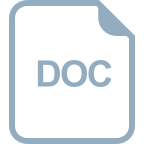




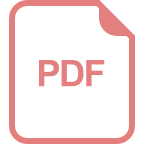
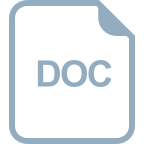
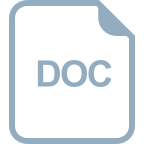


