使用 SSH 通过python 将windows 下文件夹内文件 传到阿里云服务器
时间: 2023-06-18 22:08:03 浏览: 57
您可以使用 Paramiko 库来实现在 Python 中使用 SSH 连接到阿里云服务器并传输文件。以下是一个示例代码,其中假设 Windows 文件夹的路径为 `C:\path\to\folder`,阿里云服务器的 IP 地址为 `xxx.xxx.xxx.xxx`,用户名为 `username`,密码为 `password`,远程目标文件夹的路径为 `/path/to/remote/folder`:
```python
import os
import paramiko
# Windows 文件夹路径
local_path = r"C:\path\to\folder"
# 阿里云服务器信息
host = "xxx.xxx.xxx.xxx"
port = 22
username = "username"
password = "password"
# 远程目标文件夹路径
remote_path = "/path/to/remote/folder"
# 创建 SSH 客户端
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(host, port, username, password)
# 创建 SFTP 客户端
sftp = ssh.open_sftp()
# 遍历 Windows 文件夹内的文件并传输到远程服务器
for filename in os.listdir(local_path):
local_file_path = os.path.join(local_path, filename)
if os.path.isfile(local_file_path):
remote_file_path = os.path.join(remote_path, filename)
sftp.put(local_file_path, remote_file_path)
# 关闭 SFTP 客户端和 SSH 客户端
sftp.close()
ssh.close()
```
注意,在使用 Paramiko 传输文件时,需要保证远程目标文件夹的路径已经存在。如果远程目标文件夹不存在,可以先使用 SSH 命令创建目录,例如:
```python
ssh.exec_command(f"mkdir -p {remote_path}")
```
相关推荐
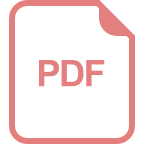
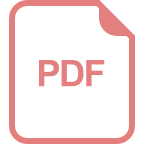
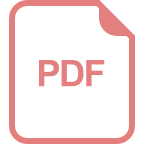














