springboot集成netty
时间: 2023-07-29 16:13:15 浏览: 112
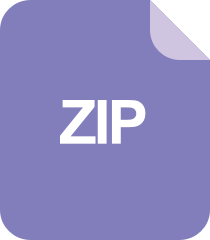
springboot与netty整合

在Spring Boot中集成Netty可以通过以下步骤完成:
1. 添加Netty依赖:在你的Spring Boot项目的pom.xml文件中添加Netty的依赖。
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.53.Final</version>
</dependency>
```
2. 创建Netty服务器:创建一个Netty服务器类,该类需要继承自`io.netty.channel.ChannelInboundHandlerAdapter`。
```java
public class NettyServer extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理接收到的消息
// ...
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 处理异常情况
// ...
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
// 在连接建立时执行一些初始化操作
// ...
}
@Override
public void channelInactive(ChannelHandlerContext ctx) {
// 在连接关闭时执行一些清理操作
// ...
}
}
```
3. 配置Netty服务器:在Spring Boot的配置文件中配置Netty服务器的相关参数,例如端口号、线程池等。
```properties
# application.properties
netty.server.port=8080
netty.server.workerThreads=10
```
4. 启动Netty服务器:在Spring Boot的启动类中初始化并启动Netty服务器。
```java
@SpringBootApplication
public class YourApplication {
@Autowired
private NettyServer nettyServer;
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
@PostConstruct
public void startNettyServer() {
// 获取Netty服务器配置参数
int port = Integer.parseInt(env.getProperty("netty.server.port"));
int workerThreads = Integer.parseInt(env.getProperty("netty.server.workerThreads"));
// 创建EventLoopGroup和ServerBootstrap
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup(workerThreads);
ServerBootstrap serverBootstrap = new ServerBootstrap();
try {
// 设置服务器参数
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 添加自定义的Handler
pipeline.addLast(nettyServer);
}
});
// 启动Netty服务器
ChannelFuture channelFuture = serverBootstrap.bind(port).sync();
channelFuture.channel().closeFuture().sync();
} catch (InterruptedException e) {
// 处理启动异常
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
这样,你就成功地在Spring Boot项目中集成了Netty。你可以在`NettyServer`类中编写自定义的业务逻辑来处理接收到的消息,并在`channelRead`方法中进行处理。同时,你也可以根据需要在`exceptionCaught`、`channelActive`和`channelInactive`方法中处理异常、连接建立和连接关闭等事件。记得在服务器关闭时进行资源的释放和清理操作。
阅读全文
相关推荐
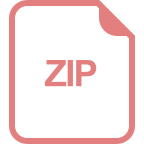
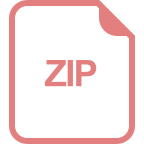
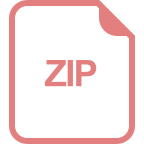
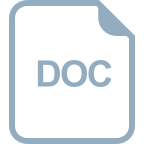








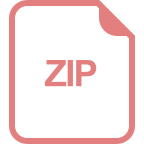
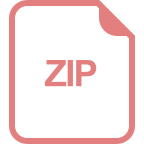
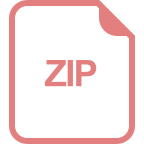

