beanutils.copyproperties替换
时间: 2024-04-06 15:25:23 浏览: 12
以下是一个替代BeanUtils.copyProperties的示例代码:
```java
import org.springframework.beans.BeanUtils;
public class BeanConverter {
public static void copyProperties(Object source, Object target) {
// 自定义的属性复制逻辑
// ...
}
}
// 使用示例
SourceBean source = new SourceBean();
TargetBean target = new TargetBean();
BeanConverter.copyProperties(source, target);
```
通过自定义的BeanConverter类,我们可以实现属性复制的逻辑。相比于BeanUtils.copyProperties,这种方式可以提供更好的性能和灵活性。
相关问题
BeanUtils.copyProperties无效
BeanUtils.copyProperties是Apache Commons BeanUtils库中的一个方法,用于将一个Java对象的属性值复制到另一个Java对象中。如果你发现BeanUtils.copyProperties方法无效,可能有以下几个原因:
1. 类型不匹配:确保源对象和目标对象的属性类型相匹配。如果属性类型不匹配,BeanUtils.copyProperties方法可能会抛出异常或者无法正确复制属性值。
2. 属性名称不匹配:确保源对象和目标对象的属性名称相匹配。如果属性名称不匹配,BeanUtils.copyProperties方法可能无法找到对应的属性进行复制。
3. 缺少getter/setter方法:确保源对象和目标对象的属性都有对应的getter和setter方法。如果缺少这些方法,BeanUtils.copyProperties方法无法访问和设置属性值。
4. 异常处理:在使用BeanUtils.copyProperties方法时,需要注意处理可能抛出的异常。例如,如果目标对象的某个属性是只读的或者没有setter方法,BeanUtils.copyProperties方法可能会抛出IllegalAccessException异常。
如果以上原因都排除了,但仍然无法解决问题,可以提供更多具体的信息和代码示例,以便更好地帮助你解决问题。
BeanUtils.copyProperties PropertyFilter
BeanUtils.copyProperties是Apache Commons BeanUtils库中的一个方法,用于将一个Java对象的属性值复制到另一个Java对象中。它可以方便地实现对象之间的属性拷贝,无需手动逐个设置属性值。
PropertyFilter是一个接口,用于在属性拷贝过程中进行过滤操作。它定义了一个方法,可以根据需要决定是否拷贝某个属性。通过实现PropertyFilter接口,可以自定义属性拷贝时的过滤规则。
以下是对BeanUtils.copyProperties和PropertyFilter的详细介绍:
1. BeanUtils.copyProperties:
- 作用:将源对象的属性值复制到目标对象中。
- 使用方法:BeanUtils.copyProperties(Object dest, Object orig)
- 参数说明:
- dest:目标对象,即要将属性值复制到的对象。
- orig:源对象,即要从中复制属性值的对象。
- 注意事项:
- 属性名和类型必须在源对象和目标对象中存在且匹配。
- 属性值会按照名称匹配进行复制,不会考虑类型转换。
2. PropertyFilter:
- 作用:在属性拷贝过程中进行过滤操作。
- 接口定义:org.apache.commons.beanutils.PropertyUtilsBean.PropertyFilter
- 方法定义:boolean apply(Object source, String name, Object value)
- 参数说明:
- source:源对象,即要从中复制属性值的对象。
- name:属性名。
- value:属性值。
- 返回值:
- true:拷贝该属性。
- false:不拷贝该属性。
相关推荐
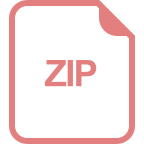
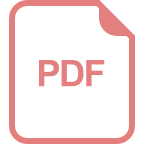
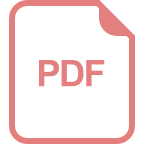












