从零开始构建Java对象工具库:Commons-BeanUtils实战手册
发布时间: 2024-09-25 14:04:21 阅读量: 114 订阅数: 44 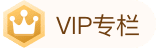
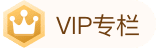
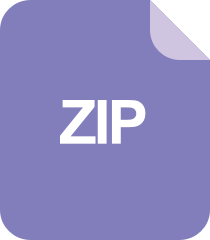
commons-beanutils-1.9.4-API文档-中文版.zip
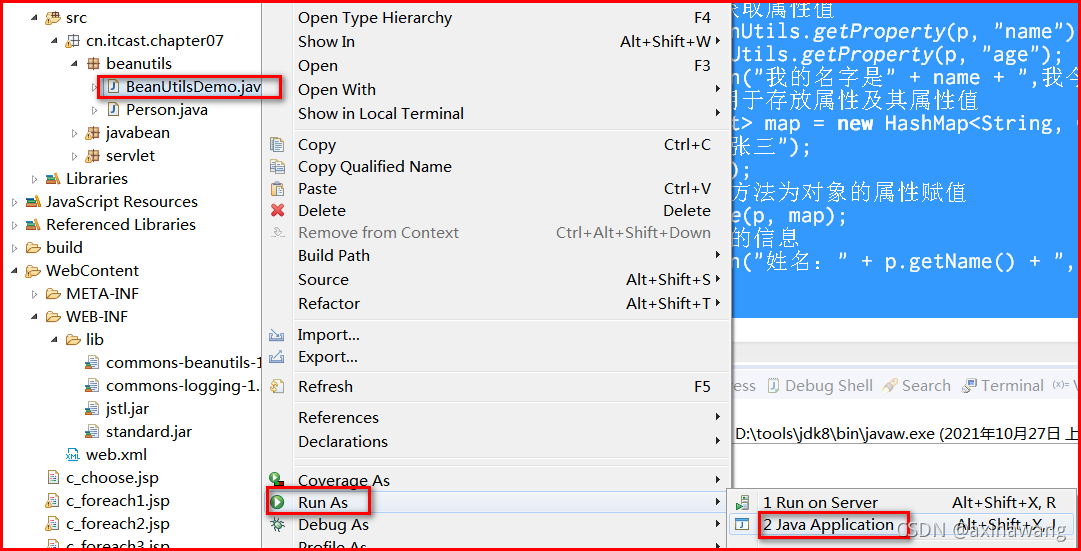
# 1. Java对象工具库简介与Commons-BeanUtils概述
Java作为当今最广泛使用的编程语言之一,拥有众多强大的库和框架,其中Java对象工具库就是其中之一。它提供了丰富的接口和工具来帮助开发者在对象之间进行数据映射和属性处理。而在众多工具库中,Commons-BeanUtils是知名度高且被广泛使用的一个。
Commons-BeanUtils是一个为Java对象操作提供了简化方式的实用库,它属于Apache Commons项目的一部分。它可以帮助开发者执行诸如对象拷贝、属性赋值、以及集合操作等常见任务。它通过使用反射机制来动态地处理对象属性,从而减少样板代码,提高开发效率。
在本文中,我们将详细介绍Commons-BeanUtils的使用和实践,包括基础功能实践、高级特性应用以及集成与扩展。随后的章节会通过代码示例和场景分析来展示如何在实际开发中运用Commons-BeanUtils,解决日常编程中遇到的常见问题。
# 2. Commons-BeanUtils基础功能实践
## 2.1 对象属性操作
### 2.1.1 获取对象属性值
在Java中,反射机制允许程序在运行时访问和操作对象的属性,而无需在编译时知道这些属性的具体信息。Commons-BeanUtils库提供了简化此类操作的API,尤其在处理对象的属性值时显得尤为方便。
使用Commons-BeanUtils获取对象属性值的基本步骤如下:
1. 首先确保已经添加了commons-beanutils库的依赖到你的项目中。
2. 创建对象实例并设置好属性值。
3. 使用`PropertyUtils.getProperty`方法获取属性值。
下面是一个简单的示例:
```***
***mons.beanutils.PropertyUtils;
public class Main {
public static void main(String[] args) throws Exception {
MyClass obj = new MyClass();
obj.setProperty("Test Value");
String value = PropertyUtils.getProperty(obj, "property");
System.out.println("The property value is: " + value);
}
public static class MyClass {
private String property;
public String getProperty() {
return property;
}
public void setProperty(String property) {
this.property = property;
}
}
}
```
在这个例子中,`PropertyUtils.getProperty`方法尝试访问`MyClass`对象`obj`的`property`属性,并返回它的值。
需要注意的是,为了能够使用`PropertyUtils.getProperty`方法,目标类中的属性必须遵循Java Bean的规范,即属性需要有对应的getter方法。
### 2.1.2 设置对象属性值
与获取属性值相对应的操作是设置对象的属性值。Commons-BeanUtils提供了`PropertyUtils.setProperty`方法来实现这一功能,其步骤与获取属性值类似:
1. 创建或获取对象实例。
2. 使用`PropertyUtils.setProperty`方法来设置属性值。
继续使用上面的例子,我们来看看如何通过Commons-BeanUtils来设置属性值:
```***
***mons.beanutils.PropertyUtils;
public class Main {
public static void main(String[] args) throws Exception {
MyClass obj = new MyClass();
PropertyUtils.setProperty(obj, "property", "New Value");
String value = PropertyUtils.getProperty(obj, "property");
System.out.println("The property value is: " + value);
}
public static class MyClass {
private String property;
public String getProperty() {
return property;
}
public void setProperty(String property) {
this.property = property;
}
}
}
```
在这个示例中,`PropertyUtils.setProperty`方法允许我们为`MyClass`对象的`property`属性赋值为"New Value"。
使用`PropertyUtils`可以显著简化反射操作,尤其是当需要操作多个对象或属性时。然而,它也带来了一定的性能开销,特别是在频繁操作属性值的场景中,因此在性能敏感的应用中需谨慎使用。
## 2.2 类型转换和数据校验
### 2.2.1 简单类型转换
Java是一种强类型语言,其类型转换通常需要明确指定,而在Web应用中,经常需要从客户端接收数据并将其转换为服务器端对象的属性。Commons-BeanUtils库中内建的类型转换器功能可以帮助我们解决这类问题。
类型转换在`PropertyUtils.setProperty`时自动进行,例如:
```***
***mons.beanutils.PropertyUtils;
import java.util.Date;
public class Main {
public static void main(String[] args) throws Exception {
MyClass obj = new MyClass();
// 字符串转换为日期类型
String dateString = "2023-03-20";
PropertyUtils.setProperty(obj, "dateProperty", dateString);
Date date = (Date) PropertyUtils.getProperty(obj, "dateProperty");
System.out.println("The date is: " + date);
}
public static class MyClass {
private Date dateProperty;
public Date getDateProperty() {
return dateProperty;
}
public void setDateProperty(Date dateProperty) {
this.dateProperty = dateProperty;
}
}
}
```
在这个例子中,Commons-BeanUtils会自动将字符串`"2023-03-20"`转换为`Date`类型的对象。
### 2.2.2 高级类型转换和自定义转换器
对于一些复杂的类型转换场景,Commons-BeanUtils允许开发者注册自定义转换器,以实现更加灵活和强大的数据处理能力。
自定义转换器需要实现`Converter`接口,例如:
```***
***mons.beanutils.Converter;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConverter implements Converter {
private SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public Object convert(Class type, Object value) {
if (value instanceof String) {
try {
return dateFormat.parse((String) value);
} catch (Exception e) {
throw new IllegalArgumentException("Date format incorrect: " + value);
}
}
throw new IllegalArgumentException("Type is not a String");
}
}
```
然后注册转换器到`conversion.properties`文件中:
```
java.util.Date=your.package.DateConverter
```
或者在代码中动态注册:
```***
***mons.beanutils.BeanUtils;
public class Main {
public static void main(String[] args) {
BeanUtils.registerConverter(Date.class, new DateConverter());
MyClass obj = new MyClass();
// 使用转换器转换字符串为日期类型
String dateString = "2023-03-20";
PropertyUtils.setProperty(obj, "dateProperty", dateString);
Date date = (Date) PropertyUtils.getProperty(obj, "dateProperty");
System.out.println("The date is: " + date);
}
public static class MyClass {
private Date dateProperty;
public Date getDateProperty() {
return dateProperty;
}
public void setDateProperty(Date dateProperty) {
this.dateProperty = dateProperty;
}
}
}
```
通过这种方式,Commons-BeanUtils在属性设置时会调用你自定义的转换器进行类型转换,从而使得类型转换更加灵活。
## 2.3 数组和集合操作
### 2.3.1 数组属性的复制
复制数组属性是Commons-BeanUtils中提供的另一项基本功能。它可以将一个数组对象的属性复制到另一个数组对象中。这个功能在处理具有数组属性的业务对象时非常有用。
复制数组属性的一个基本步骤是:
1. 创建源数组和目标数组。
2. 调用`ArrayUtils.copyArray`方法来复制数组。
下面是一个使用Commons-BeanUtils复制数组属性的示例:
```***
***mons.beanutils.ArrayUtils;
public class Main {
public static void main(String[] args) {
// 创建源数组
MyClass[] sources = new MyClass[2];
sources[0] = new MyClass("First");
sources[1] = new MyClass("Second");
// 创建目标数组
MyClass[] targets = new MyClass[2];
// 复制数组
ArrayUtils.copyArray(targets, sources);
// 输出目标数组的内容,验证复制是否成功
for (MyClass target : targets) {
System.out.println(target);
}
}
public static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
@Override
public String toString() {
return this.name;
}
}
}
```
在上述代码中,通过`ArrayUtils.copyArray`方法将`sources`数组复制到了`targets`数组中。这种方法特别适用于需要动态复制数组属性的场景,例如在数据传输对象(DTO)转换等场景中。
### 2.3.2 集合对象的处理方法
Commons-BeanUtils库不仅支持数组的复制,还提供了集合对象的复制方法。`CollectionUtils.copyCollection`方法可以将一个集合对象的元素复制到另一个集合对象中。
假设我们有一个列表,我们希望将其内容复制到另一个列表中,可以使用下面的方法:
```***
***mons.beanutils.CollectionUtils;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// 创建源集合
List<String> sourceList = new ArrayList<>();
sourceList.add("On
```
0
0
相关推荐
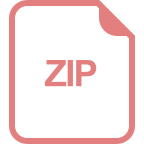
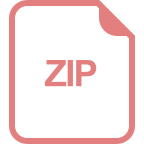
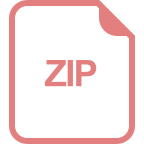
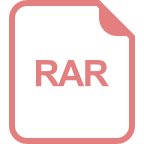
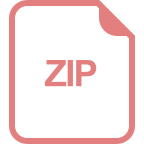
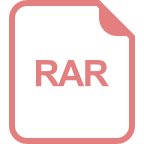
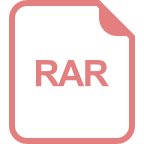
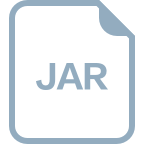