职工考勤管理系统c++
时间: 2023-11-30 18:41:59 浏览: 47
以下是一个简单的职工考勤管理系统的C++代码示例,其中包括员工信息管理、考勤管理、统计查询和用户管理等模块:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
// 定义员工类
class Employee {
public:
Employee(int id, string name, string gender, string department, string level) {
this->id = id;
this->name = name;
this->gender = gender;
this->department = department;
this->level = level;
}
int getId() { return id; }
string getName() { return name; }
string getGender() { return gender; }
string getDepartment() { return department; }
string getLevel() { return level; }
private:
int id;
string name;
string gender;
string department;
string level;
};
// 定义考勤类
class Attendance {
public:
Attendance(Employee* employee, int year, int month, int day, int hour, int minute, string type) {
this->employee = employee;
this->year = year;
this->month = month;
this->day = day;
this->hour = hour;
this->minute = minute;
this->type = type;
}
Employee* getEmployee() { return employee; }
int getYear() { return year; }
int getMonth() { return month; }
int getDay() { return day; }
int getHour() { return hour; }
int getMinute() { return minute; }
string getType() { return type; }
private:
Employee* employee;
int year;
int month;
int day;
int hour;
int minute;
string type;
};
// 定义节假日类
class Holiday {
public:
Holiday(int year, int month, int day, string name) {
this->year = year;
this->month = month;
this->day = day;
this->name = name;
}
int getYear() { return year; }
int getMonth() { return month; }
int getDay() { return day; }
string getName() { return name; }
private:
int year;
int month;
int day;
string name;
};
// 定义部门类
class Department {
public:
Department(int id, string name) {
this->id = id;
this->name = name;
}
int getId() { return id; }
string getName() { return name; }
private:
int id;
string name;
};
// 定义用户类
class User {
public:
User(string username, string password, string role) {
this->username = username;
this->password = password;
this->role = role;
}
string getUsername() { return username; }
string getPassword() { return password; }
string getRole() { return role; }
private:
string username;
string password;
string role;
};
// 定义抽象类
class AbstractClass {
public:
virtual void add() = 0;
virtual void remove() = 0;
virtual void update() = 0;
virtual void query() = 0;
};
// 定义员工信息管理类
class EmployeeManager : public AbstractClass {
public:
void add() {
// 添加员工信息
}
void remove() {
// 删除员工信息
}
void update() {
// 更新员工信息
}
void query() {
// 查询员工信息
}
};
// 定义考勤管理类
class AttendanceManager : public AbstractClass {
public:
void add() {
// 添加考勤信息
}
void remove() {
// 删除考勤信息
}
void update() {
// 更新考勤信息
}
void query() {
// 查询考勤信息
}
};
// 定义统计查询类
class StatisticManager : public AbstractClass {
public:
void add() {
// 添加统计信息
}
void remove() {
// 删除统计信息
}
void update() {
// 更新统计信息
}
void query() {
// 查询统计信息
}
};
// 定义用户管理类
class UserManager : public AbstractClass {
public:
void add() {
// 添加用户信息
}
void remove() {
// 删除用户信息
}
void update() {
// 更新用户信息
}
void query() {
// 查询用户信息
}
};
// 定义文件读写类
class FileUtil {
public:
static vector<string> read(string filename) {
vector<string> lines;
ifstream fin(filename);
if (fin) {
string line;
while (getline(fin, line)) {
lines.push_back(line);
}
fin.close();
}
return lines;
}
static void write(string filename, vector<string> lines) {
ofstream fout(filename);
if (fout) {
for (string line : lines) {
fout << line << endl;
}
fout.close();
}
}
};
// 定义系统类
class System {
public:
System() {
// 初始化系统
}
void login(string username, string password) {
// 用户登录
}
void logout() {
// 用户退出
}
void run() {
// 运行系统
}
private:
vector<Employee*> employees;
vector<Attendance*> attendances;
vector<Holiday*> holidays;
vector<Department*> departments;
vector<User*> users;
};
int main() {
System system;
system.run();
return 0;
}
```
相关推荐
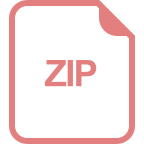
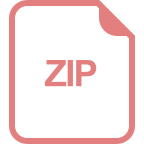














