9.从任意新闻网站下载100条的新闻数据,并输出。特别要收集标题、内容、时间、作者这四个信息。 ① 请求单个网页 ② 对单个页面进行数据的提取(方法任选:可以正则表达式、beautifulsoap) ③ 找到一个新闻的链接页,请求新闻列表,并使用
时间: 2024-09-10 12:23:57 浏览: 60
抓取网络新闻数据涉及到网络爬虫技术,特别是针对HTML结构的数据。以下是步骤概述:
1. **请求单个网页**:
- 使用Python的`requests`库来发送HTTP请求,获取网页源码。
```python
import requests
url = 'http://example.com/news' # 需要替换为你想抓取的新闻网站的URL
response = requests.get(url)
```
2. **数据提取**:
- 可以通过BeautifulSoup库解析HTML文档,定位并提取所需的信息(标题、内容、时间、作者)。这里以BeautifulSoup为例:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h2') # 假设标题在<h2>标签内
contents = [p.get_text() for p in soup.find_all('p')] # 查找所有<p>元素的内容
timestamps = [td.get_text() for td in soup.find_all('time')] # 找到<time>元素的时间
authors = soup.find_all(class_='author') # 类名假设是'author'
```
3. **遍历和获取新闻列表**:
- 如果每个新闻是一系列链接,可以使用`response.links`属性来查找新闻列表。然后递归地访问每一条链接。
```python
links = response.html.select('.news-list a') # 假设链接在CSS选择器'.news-list a'中
for link in links:
link_page_url = link['href']
# 递归请求链接页面并提取数据
```
4. **存储数据**:
- 抓取的数据需要保存起来,你可以使用CSV文件、数据库或者JSON文件等,比如`csv`模块:
```python
import csv
with open('news_data.csv', 'a', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow([title, content, timestamp, author])
```
请注意,实际操作时要遵守网站的robots.txt协议以及尊重版权。此外,频繁抓取可能会导致IP被封禁,所以最好设置合理的延时。
阅读全文
相关推荐
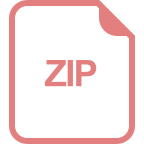
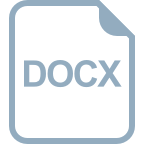
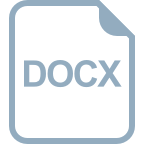

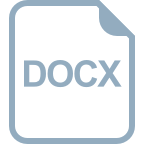
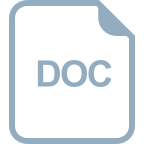
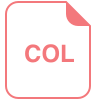
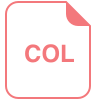
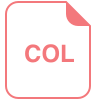
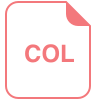
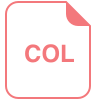
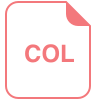
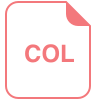
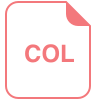
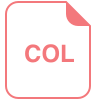
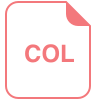
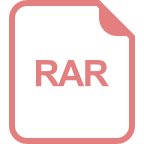
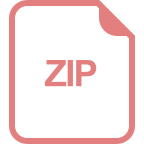