std::string 剔除指定字符
时间: 2024-05-27 20:05:52 浏览: 78
可以使用erase和remove算法来剔除std::string中的指定字符。下面是一些示例代码:
1. 使用std::erase和std::remove
```
std::string str = "hello, world!";
char ch = ',';
str.erase(std::remove(str.begin(), str.end(), ch), str.end());
// 最终结果为"hello world!"
```
2. 使用自定义函数
```
void removeChar(std::string& str, char ch) {
str.erase(std::remove(str.begin(), str.end(), ch), str.end());
}
// 调用
std::string str = "hello, world!";
removeChar(str, ',');
// 最终结果为"hello world!"
```
3. 使用lambda表达式
```
std::string str = "hello, world!";
char ch = ',';
str.erase(std::remove_if(str.begin(), str.end(), [&](char c){ return c == ch; }), str.end());
// 最终结果为"hello world!"
```
阅读全文
相关推荐
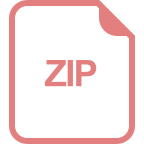
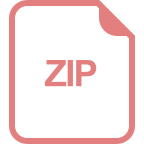
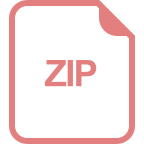
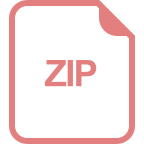
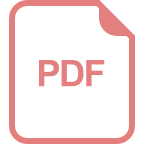
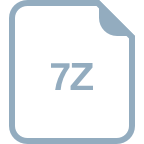
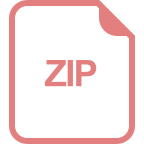
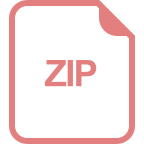
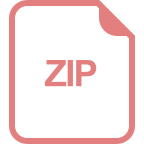
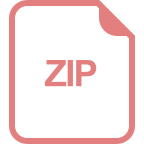
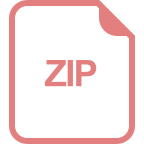
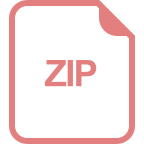
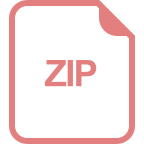
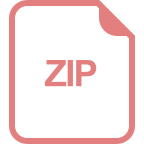