c++判断某个数是否在vector中
时间: 2024-09-06 21:06:35 浏览: 20
在 C++ 中,判断一个数是否在一个 `std::vector` 中,通常可以使用 `std::find()` 函数或者直接遍历整个容器。以下是两种方法的示例:
**方法一:使用 find() 函数**
```cpp
#include <algorithm>
#include <vector>
bool isInVector(int num, std::vector<int>& vec) {
auto it = std::find(vec.begin(), vec.end(), num);
return (it != vec.end());
}
```
这里 `std::find()` 如果找到元素则返回对应的迭代器,如果没找到则返回 `vec.end()`。所以通过比较迭代器位置是否到达结束位置,就可以判断数字是否在 vector 中。
**方法二:遍历整个向量**
```cpp
bool isInVector(int num, std::vector<int>& vec) {
for (int value : vec) {
if (value == num) {
return true;
}
}
return false;
}
```
这个方法直接遍历整个 vector,遇到相同的数字就返回 true。
相关问题
用c++编写一个在vector容器中判断某个数是否存在的代码
以下是用 C++ 编写一个在 vector 容器中判断某个数是否存在的代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
int target = 3;
if (find(nums.begin(), nums.end(), target) != nums.end()) {
cout << "Target exists in the vector." << endl;
} else {
cout << "Target does not exist in the vector." << endl;
}
return 0;
}
```
这段代码中,我们首先定义了一个 vector 容器 nums,其中包含了一些整数。然后我们定义了一个目标数 target,我们需要判断这个数是否存在于 nums 中。
我们使用了 C++ 标准库中的 find 函数来查找 target 是否存在于 nums 中。如果 find 函数返回的迭代器不等于 nums.end(),则说明 target 存在于 nums 中,否则说明 target 不存在于 nums 中。
注意:这段代码只是一个示例,实际使用时需要根据具体情况进行修改。
c++ 如何判断vector中是否半酣某个元素
在 C++ 中,可以使用 `find()` 函数来查找 `vector` 中是否包含某个元素。该函数的用法如下:
```c++
#include <vector>
#include <algorithm>
using namespace std;
// 判断 vector 中是否包含某个元素
bool isContain(vector<int>& vec, int num) {
return (find(vec.begin(), vec.end(), num) != vec.end());
}
```
这里定义了一个名为 `isContain()` 的函数,该函数接受一个 `vector` 和一个整数作为参数,用于判断该 `vector` 是否包含该整数。函数使用 STL 中的 `find()` 函数来查找元素,如果找到了该元素,则返回 `true`,否则返回 `false`。
以下是一个示例程序,演示了如何使用 `isContain()` 函数来判断 `vector` 中是否包含某个元素:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool isContain(vector<int>& vec, int num) {
return (find(vec.begin(), vec.end(), num) != vec.end());
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5}; // 定义 vector
int num = 3; // 要查找的元素
if (isContain(vec, num)) {
cout << "vector 中包含元素 " << num << endl;
} else {
cout << "vector 中不包含元素 " << num << endl;
}
return 0;
}
```
运行程序后,屏幕上会输出 `vector 中包含元素 3`。如果将 `num` 的值修改为 6,则会输出 `vector 中不包含元素 6`。
相关推荐
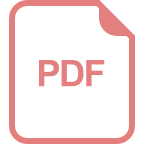
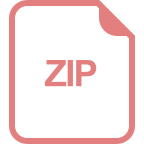
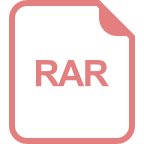












