判断vector容器里面是否存在某个值
时间: 2023-09-12 10:08:04 浏览: 97
你可以使用`std::find`算法函数来判断vector容器里面是否存在某个值。std::find函数会在指定的范围内查找给定值,并返回指向该值的迭代器,如果找不到,则返回指向容器末尾的迭代器。以下是一个示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
int main()
{
std::vector<int> vec = {1, 2, 3, 4, 5};
int value = 3;
auto it = std::find(vec.begin(), vec.end(), value);
if(it != vec.end())
{
std::cout << "Value " << value << " found in vector" << std::endl;
}
else
{
std::cout << "Value " << value << " not found in vector" << std::endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个名为vec的vector容器,并使用std::find算法函数来查找值为3的元素是否存在于vec中。如果找到了该元素,则打印消息“Value 3 found in vector”,否则打印“Value 3 not found in vector”。
相关问题
vector内是否存在某个值
可以使用 STL 中的 `std::find` 函数来判断一个 `vector` 容器内是否存在某个值,示例如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int target = 3;
auto it = std::find(vec.begin(), vec.end(), target);
if (it != vec.end()) {
std::cout << "Target found in vector." << std::endl;
} else {
std::cout << "Target not found in vector." << std::endl;
}
return 0;
}
```
其中,`std::find` 函数返回一个迭代器,指向第一个等于目标值的元素,如果没有找到,则返回指向容器末尾的迭代器 `vec.end()`。通过判断返回的迭代器是否等于 `vec.end()`,即可确定容器内是否存在目标值。
用c++编写一个在vector容器中判断某个数是否存在的代码
以下是用 C++ 编写一个在 vector 容器中判断某个数是否存在的代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
int target = 3;
if (find(nums.begin(), nums.end(), target) != nums.end()) {
cout << "Target exists in the vector." << endl;
} else {
cout << "Target does not exist in the vector." << endl;
}
return 0;
}
```
这段代码中,我们首先定义了一个 vector 容器 nums,其中包含了一些整数。然后我们定义了一个目标数 target,我们需要判断这个数是否存在于 nums 中。
我们使用了 C++ 标准库中的 find 函数来查找 target 是否存在于 nums 中。如果 find 函数返回的迭代器不等于 nums.end(),则说明 target 存在于 nums 中,否则说明 target 不存在于 nums 中。
注意:这段代码只是一个示例,实际使用时需要根据具体情况进行修改。
阅读全文
相关推荐
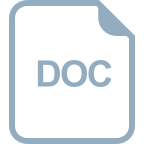
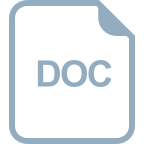
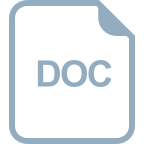
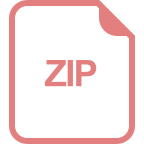
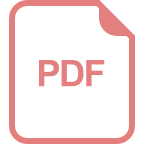
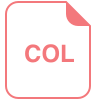










