cpp从类容器中删除具有某个属性值的元素
时间: 2023-07-26 20:13:07 浏览: 97
假设你的类容器是一个 `vector`,你希望删除其中所有满足某个属性的元素,可以使用以下代码:
首先,你需要定义一个谓词(predicate)函数,用于判断元素是否满足条件。假设你的类为 `MyClass`,属性为 `MyProperty`,你可以定义如下谓词函数:
```
bool MyPredicate(const MyClass& obj) {
return obj.MyProperty == some_value;
}
```
然后,你可以使用 `remove_if` 算法来移动所有满足条件的元素到容器末尾:
```
vector<MyClass> myVector; // 假设 myVector 已经填充了一些元素
// 移动所有满足条件的元素到容器末尾
myVector.erase(remove_if(myVector.begin(), myVector.end(), MyPredicate), myVector.end());
```
上述代码中,`remove_if` 算法会遍历容器中的元素,将所有满足条件的元素移动到容器的末尾,然后返回指向第一个被移动元素的迭代器。然后,`erase` 成员函数会将末尾的这些元素从容器中删除。
相关问题
cpp从类容器中删除指定属性值的元素
假设你的类容器是一个 `vector`,你希望删除其中所有值为 `x` 的元素,可以使用以下代码:
```
vector<int> myVector; // 假设 myVector 已经填充了一些元素
// 删除所有等于 x 的元素
myVector.erase(remove(myVector.begin(), myVector.end(), x), myVector.end());
```
上述代码中,`remove` 算法会将所有值为 `x` 的元素移动到容器的末尾,然后返回指向第一个被移动元素的迭代器。然后,`erase` 成员函数会将末尾的这些元素从容器中删除。
cpp删除类容器中容器属性元素
在C++中删除类容器中容器属性元素的方法与其他容器类型类似。以下是一个删除嵌套vector中元素的示例代码:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> myVector{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int elementToRemove = 5;
// Loop through each vector in the outer vector
for (auto& innerVector : myVector) {
// Use an iterator to loop through each element in the inner vector
for (auto it = innerVector.begin(); it != innerVector.end(); ++it) {
if (*it == elementToRemove) {
// Erase the element from the inner vector
innerVector.erase(it);
break; // Break out of the loop once the element is removed
}
}
}
// Print the updated vector
for (auto& innerVector : myVector) {
for (auto& element : innerVector) {
std::cout << element << " ";
}
std::cout << std::endl;
}
return 0;
}
```
在这个示例中,我们使用双重循环来遍历外层和内层vector中的元素。内层循环使用迭代器访问每个元素,如果找到了要删除的元素,则使用erase方法从vector中删除该元素。需要注意的是,我们使用break语句来退出内层循环,这是因为erase方法会使迭代器失效,如果不退出循环,会导致未定义行为。
阅读全文
相关推荐
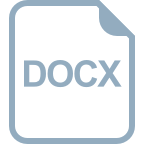
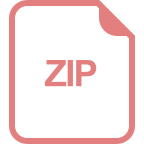
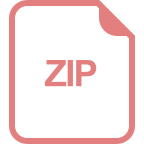













