lpctstr转string
时间: 2023-08-16 22:12:39 浏览: 74
要将LPCTSTR转换为string,您可以使用以下代码示例:
```cpp
#include <string>
#include <Windows.h>
std::string LPCTSTRToString(LPCTSTR lpszText) {
int length = lstrlen(lpszText);
std::string str(lpszText, length);
return str;
}
int main() {
LPCTSTR lpszText = _T("Hello, World!"); // 假设有一个LPCTSTR字符串
std::string result = LPCTSTRToString(lpszText);
// 现在,result包含了转换后的字符串
return 0;
}
```
在上面的示例中,`LPCTSTRToString`函数将LPCTSTR字符串转换为std::string。它使用`lstrlen`函数获取字符串长度,并通过std::string构造函数将LPCTSTR字符串转换为std::string对象。最后,它返回转换后的字符串。
请注意,上述示例假设您正在使用Windows操作系统,并且已经包含了Windows.h头文件。如果您是在其他操作系统或环境中使用LPCTSTR,请相应地调整代码。
相关问题
Unicode 编译环境中,LPCTSTR 转string
在 Unicode 编译环境中,`LPCTSTR` 是指向宽字符的指针,即 `const wchar_t*` 类型的指针。要将 `LPCTSTR` 转换为 `std::string`,需要进行一些额外的步骤。
下面是一个示例代码,演示如何将 `LPCTSTR` 转换为 `std::string`:
```cpp
#include <string>
#include <locale>
#include <codecvt>
std::string LPCTSTRToString(LPCTSTR lpctstr) {
std::wstring_convert<std::codecvt_utf8_utf16<wchar_t>> converter;
std::wstring wstr(lpctstr);
return converter.to_bytes(wstr);
}
```
这里使用了 `std::wstring_convert` 和 `std::codecvt_utf8_utf16` 进行宽字符到 UTF-8 字符串的转换。首先,将 `LPCTSTR` 转换为 `std::wstring`,然后使用 `std::wstring_convert` 将宽字符串转换为 UTF-8 字符串。
使用上述示例代码,你可以调用 `LPCTSTRToString` 函数将 `LPCTSTR` 转换为 `std::string`。例如:
```cpp
LPCTSTR lpctstr = L"Hello, world!";
std::string str = LPCTSTRToString(lpctstr);
```
现在,`str` 将包含转换后的 UTF-8 字符串。请注意,这个示例假设你的编译器和运行环境支持 C++11 或更高版本的标准库。
我的编译器和运行环境不支持 C++11 ,如何在Unicode 编译环境中,LPCTSTR 转string
如果你的编译器和运行环境不支持 C++11 或更高版本的标准库,你可以使用 Windows API 提供的函数来进行 `LPCTSTR` 到 `std::string` 的转换。
下面是一个示例代码,演示如何在 Unicode 编译环境中将 `LPCTSTR` 转换为 `std::string`:
```cpp
#include <string>
#include <windows.h>
std::string LPCTSTRToString(LPCTSTR lpctstr) {
int length = WideCharToMultiByte(CP_UTF8, 0, lpctstr, -1, nullptr, 0, nullptr, nullptr);
std::string str(length - 1, '\0');
WideCharToMultiByte(CP_UTF8, 0, lpctstr, -1, &str[0], length, nullptr, nullptr);
return str;
}
```
这里使用了 `WideCharToMultiByte` 函数将宽字符转换为 UTF-8 字符串。首先,调用一次 `WideCharToMultiByte` 获取转换后字符串的长度,然后创建一个足够容纳转换后字符串的 `std::string` 对象。最后,再次调用 `WideCharToMultiByte` 进行实际的转换。
使用上述示例代码,你可以调用 `LPCTSTRToString` 函数将 `LPCTSTR` 转换为 `std::string`。例如:
```cpp
LPCTSTR lpctstr = L"Hello, world!";
std::string str = LPCTSTRToString(lpctstr);
```
现在,`str` 将包含转换后的 UTF-8 字符串。
请注意,这种方法依赖于 Windows API,并且在 Windows 平台上才适用。
相关推荐
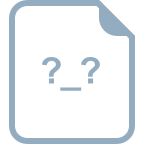
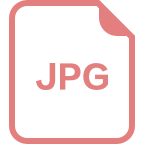












