Python画图柱状图
时间: 2023-11-05 15:22:23 浏览: 91
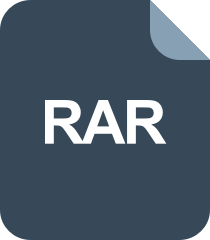
1_matplotlib_python画图_
当使用Python绘制柱状图时,可以使用matplotlib库来完成。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
# 柱状图的数据
x = ['A', 'B', 'C', 'D', 'E']
y = [10, 20, 15, 25, 30]
# 创建柱状图
plt.bar(x, y)
# 设置标题和标签
plt.title('柱状图示例')
plt.xlabel('类别')
plt.ylabel('数值')
# 显示图形
plt.show()
```
在示例代码中,我们定义了一个包含类别和对应数值的数据列表。然后使用`plt.bar()`函数创建柱状图,其中`x`表示类别,`y`表示对应的数值。
接下来,我们使用`plt.title()`、`plt.xlabel()`和`plt.ylabel()`函数设置标题和标签。
最后,使用`plt.show()`函数显示绘制出来的柱状图。
你可以根据自己的需求修改数据和图形样式。希望这个示例对你有帮助!
阅读全文
相关推荐
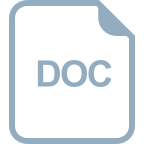
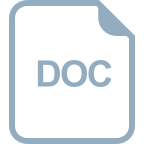
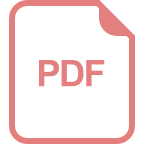


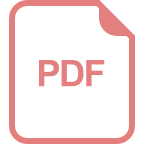
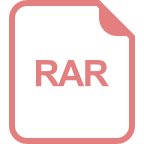










