'XWPFDocument' used without 'try'-with-resources statement
时间: 2024-01-05 16:02:12 浏览: 22
你在使用 'XWPFDocument' 时没有使用 'try'-with-resources 语句。这可能会导致资源泄漏或文件未正确关闭。在使用 'XWPFDocument' 时,建议使用 'try'-with-resources 语句来自动关闭文件。示例如下:
```java
try (XWPFDocument document = new XWPFDocument(new FileInputStream("example.docx"))) {
// 在这里处理你的文档操作
} catch (IOException e) {
// 处理异常
}
```
通过这种方式,当代码块执行完毕或发生异常时,'XWPFDocument' 对象会被自动关闭,无需手动调用 'close()' 方法。这样可以确保资源的正确释放,避免潜在的问题。
相关问题
'KafkaConsumer<String, String>' used without 'try'-with-resources statement
这个警告是因为 `KafkaConsumer` 实现了 `java.lang.AutoCloseable` 接口,需要使用 try-with-resources 语句来自动关闭 `KafkaConsumer` 实例。
使用 try-with-resources 语句可以自动关闭 `KafkaConsumer` 实例,避免资源泄露。例如:
```java
try (KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props)) {
consumer.subscribe(Arrays.asList("topic1", "topic2"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("offset = %d, key = %s, value = %s%n", record.offset(), record.key(), record.value());
}
}
} catch (Exception e) {
e.printStackTrace();
}
```
在这个例子中,我们使用 try-with-resources 语句来创建 `KafkaConsumer` 实例,并在 try 块结束时自动调用 `close` 方法关闭实例。这样可以避免资源泄露,并且代码更加简洁。如果在 try 块中发生异常,也不需要手动关闭 `KafkaConsumer` 实例,它会自动被关闭。
需要注意的是,在使用 try-with-resources 语句时,需要将 `KafkaConsumer` 实例的声明放在 try-with-resources 语句的括号中,确保在 try 块结束时自动关闭实例。同时,需要捕获异常,避免异常导致程序崩溃。
e: there are problems and -y was used without --force-yes
This is because the usage of `-y` without `--force-yes` can cause issues in package installation and upgrades. The correct way to use `apt-get` with automatic yes to prompts is to use the `-y` flag along with the `--allow-downgrades` flag, like so:
```
sudo apt-get upgrade -y --allow-downgrades
```
This will allow automatic yes to prompts while also allowing downgrades if necessary.
相关推荐
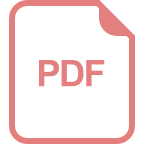












