二叉树的遍历递归算法
时间: 2023-11-20 09:54:26 浏览: 114
二叉树的遍历递归算法是指通过递归的方式,按照一定的顺序遍历二叉树中的所有节点。常见的遍历方式有前序遍历、中序遍历和后序遍历。具体实现方法如下:
前序遍历:先访问根节点,再访问左子树,最后访问右子树。代码实现如下:
```python
def preorderTraversal(root):
if not root:
return []
res = []
res.append(root.val)
res += preorderTraversal(root.left)
res += preorderTraversal(root.right)
return res
```
中序遍历:先访问左子树,再访问根节点,最后访问右子树。代码实现如下:
```python
def inorderTraversal(root):
if not root:
return []
res = []
res += inorderTraversal(root.left)
res.append(root.val)
res += inorderTraversal(root.right)
return res
```
后序遍历:先访问左子树,再访问右子树,最后访问根节点。代码实现如下:
```python
def postorderTraversal(root):
if not root:
return []
res = []
res += postorderTraversal(root.left)
res += postorderTraversal(root.right)
res.append(root.val)
return res
```
阅读全文
相关推荐





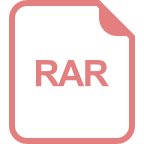
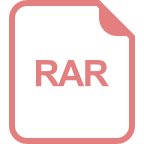
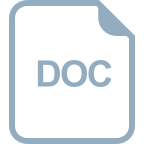
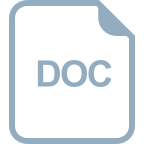
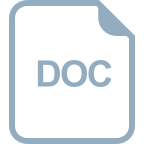



