vs平台用c语言写串口调用部分
时间: 2024-10-09 10:01:44 浏览: 15
在VS平台上使用C语言编写串口通信的部分通常涉及到几个步骤:
1. **包含头文件**:首先,你需要包含相关的串口通信库,比如`stdio.h`、`fcntl.h`、`termios.h`等,它们提供了控制终端输入输出的功能。
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
```
2. **打开串口**:通过`open()`函数打开串口设备,例如 `/dev/ttyS0`(取决于你的系统),设置访问权限和标志。
```c
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Failed to open serial port");
}
```
3. **配置串口**:使用`tcgetattr()`获取当前的终端属性,然后修改`struct termios`结构体来设定波特率、数据位、停止位和奇偶校验等参数,再用`tcsetattr()`保存更改。
```c
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600 bps
cfsetospeed(&options, B9600); // 设置传输速度与接收速度一致
...
tcflush(fd, TCIFLUSH); // 清空缓冲区
tcsetattr(fd, TCSANOW, &options); // 应用新的设置
```
4. **发送和接收数据**:你可以使用`write()`函数发送数据到串口,用`read()`函数从串口读取数据。
```c
char data[256];
memset(data, 0, sizeof(data));
// 发送数据
write(fd, "Hello from C!", strlen("Hello from C!") + 1);
// 接收数据
ssize_t bytes_received = read(fd, data, sizeof(data));
if (bytes_received > 0) {
printf("Received: %s\n", data);
}
```
5. **关闭串口**:完成操作后记得关闭串口资源。
```c
close(fd);
```
阅读全文
相关推荐
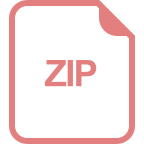
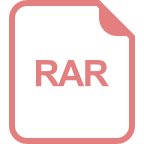
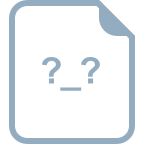
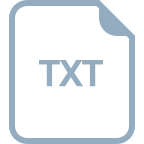
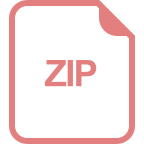
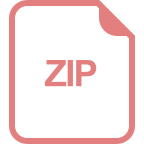
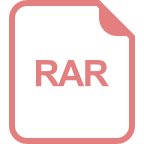
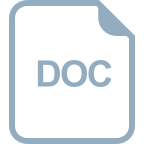
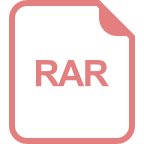
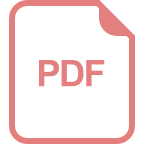
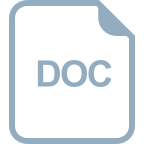
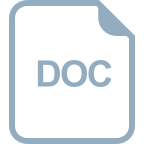
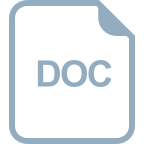





