实战项目 爬取京东商城网站数据 任务: 京东商城有大量的商品数据,在搜索框中输入某类商品,例如"手机",就可以看到近百 页手机的信息。现在我们使用 Selenium 编写一个爬虫程序,自动在输入框输入"手机",自动 翻页爬取所有手机的数据与图像,并保存到数据库
时间: 2024-12-23 19:20:23 浏览: 11
实战项目:爬取京东商城网站数据通常涉及使用网络爬虫技术,如Selenium配合Python等工具来自动化获取网页信息。以下是这个任务的大致步骤:
1. **安装必要的库**:
- 首先,需要安装`selenium`用于浏览器控制、`webdriver_manager`用于管理浏览器驱动、`requests`处理HTTP请求以及`BeautifulSoup`解析HTML文档。
```
pip install selenium webdriver_manager requests beautifulsoup4
```
2. **配置浏览器驱动**:
- 下载对应的浏览器驱动(如ChromeDriver),并将其路径添加到环境变量中。
3. **编写爬虫脚本**:
```python
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
from bs4 import BeautifulSoup
import time
def get_jd_data(keyword):
# 初始化浏览器
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
url = "https://search.jd.com/Search?keyword=" + keyword
driver.get(url)
# 翻页函数
def scroll_to_bottom():
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(1)
new_height = driver.execute_script("return document.body.scrollHeight")
if new_height == last_height:
break
last_height = new_height
# 获取页面内容
soup = BeautifulSoup(driver.page_source, 'lxml')
products = soup.find_all('div', class_='gl-item')
# 提取所需数据(如商品名、价格、图片URL等)
data = []
for product in products:
title = product.find('a', class_='p-name').text
price = product.find('span', class_='p-price').text
image_url = product.find('img', class_='lazy')['data-lazy-src']
data.append({'title': title, 'price': price, 'image_url': image_url})
# 保存到数据库
save_to_db(data)
# 自动翻页,直到没有更多结果
while True:
scroll_to_bottom()
next_button = driver.find_element_by_css_selector('.gl-pager-next a')
if not next_button.is_displayed() or next_button.get_attribute('disabled'):
break
next_button.click()
def save_to_db(data):
# 这里需要连接到数据库(如MySQL、MongoDB等),并将数据插入相应表中
pass
# 主函数
if __name__ == "__main__":
keyword = "手机"
get_jd_data(keyword)
```
4. **数据持久化与安全注意事项**:
- 数据需要存储到数据库,可以使用ORM框架如SQLAlchemy或MongoEngine简化操作。
- 为了遵守京东的反爬虫策略,应设置合理的延时(使用time.sleep)并随机化请求间隔。
- 关注robots.txt文件,尊重网站抓取规则。
阅读全文
相关推荐
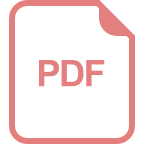
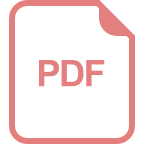
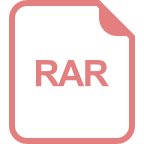
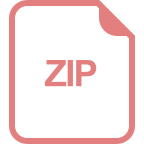
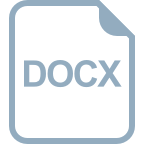
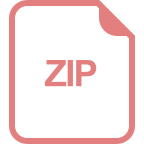
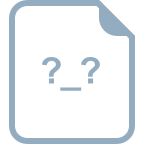
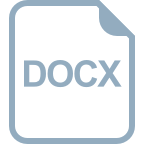
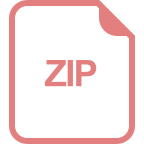
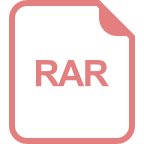
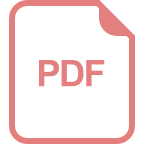
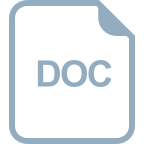
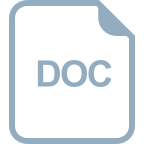
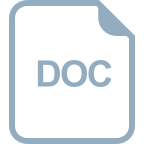
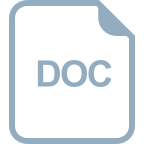
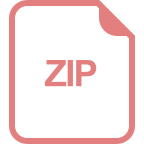