wpf 窗口遮住windows工具栏
时间: 2023-08-12 13:09:29 浏览: 50
在 WPF 中,如果要使窗口遮住 Windows 任务栏,可以通过以下步骤来实现:
1. 在 Window 对象的 XAML 中,设置 WindowStyle 属性为 None,这将隐藏窗口的标题栏和边框。
```xaml
<Window x:Class="YourNamespace.YourWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Your Window" WindowStyle="None">
<!-- Window Content -->
</Window>
```
2. 在 Window 对象的 XAML 中,设置 WindowState 属性为 Maximized,这将使窗口最大化。
```xaml
<Window x:Class="YourNamespace.YourWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Your Window" WindowStyle="None" WindowState="Maximized">
<!-- Window Content -->
</Window>
```
3. 在代码中,调用 Win32 API 来隐藏任务栏。首先,创建一个名为 "NativeMethods" 的类,并导入以下命名空间:
```csharp
using System;
using System.Runtime.InteropServices;
```
4. 在 "NativeMethods" 类中,定义一个名为 "ShowWindow" 的静态方法,用于调用 Win32 API 来显示或隐藏任务栏:
```csharp
public static class NativeMethods
{
[DllImport("user32.dll")]
public static extern int FindWindow(string lpClassName, string lpWindowName);
[DllImport("user32.dll")]
public static extern int ShowWindow(int hwnd, int nCmdShow);
}
```
5. 在窗口的 Loaded 事件处理程序中,调用 "NativeMethods.ShowWindow" 方法来隐藏任务栏。在这之前,需要获取任务栏的窗口句柄并传递给 ShowWindow 方法:
```csharp
private void Window_Loaded(object sender, RoutedEventArgs e)
{
int hwnd = NativeMethods.FindWindow("Shell_TrayWnd", "");
NativeMethods.ShowWindow(hwnd, 0); // 0 表示隐藏任务栏
}
```
这样,当窗口加载时,它将以全屏模式显示,并且会遮住 Windows 任务栏。请注意,这种方法需要使用 P/Invoke 调用 Win32 API,因此需要添加相应的命名空间和引用。
相关推荐
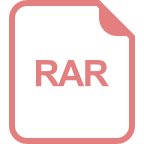
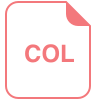
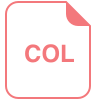
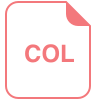











