如何实现一个基于Vue前端和SpringBoot后端的简单前后端分离项目,包括增删改查功能的前后端代码示例?
时间: 2024-10-16 18:22:05 浏览: 39
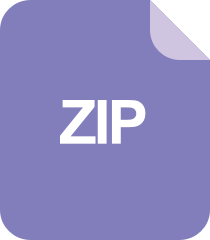
Java毕业设计-基于SpringBoot+Vue+ElementUI 前后端分离的人力资源管理系统(源码+数据库脚本).zip

创建一个基于Vue前端和SpringBoot后端的简单前后端分离项目,可以按照以下步骤来进行:
### 前端Vue:
首先安装Vue CLI(Vue脚手架):
```bash
npm install -g @vue/cli
```
然后创建一个新的Vue项目:
```bash
vue create my-project
cd my-project
```
接下来,在`src`目录下创建组件,比如`Item.vue`来表示商品信息:
```html
<template>
<div>
<input v-model="item.name" placeholder="名称">
<input v-model="item.price" type="number" placeholder="价格">
<button @click="addItem()">添加</button>
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item.name }} - {{ item.price }}
<button @click="deleteItem(index)">删除</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
item: { name: '', price: '' },
items: [],
};
},
methods: {
addItem() {
this.items.push(this.item);
this.item = {};
},
deleteItem(index) {
this.items.splice(index, 1);
},
},
};
</script>
```
### 后端SpringBoot:
1. 首先创建SpringBoot项目:
```sh
spring init -d web,jpa my-backend
```
2. 在`application.properties`中配置Spring Data JPA:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
```
3. 定义一个Repository接口,例如`ItemRepository`:
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface ItemRepository extends JpaRepository<Item, Long> {}
```
4. 创建一个`Item`实体类:
```java
public class Item {
private Long id;
private String name;
private Double price;
// getters and setters
}
```
5. `Controller`中处理HTTP请求:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
public class ItemController {
@Autowired
private ItemRepository repository;
@PostMapping("/items")
public Item create(@RequestBody Item item) {
return repository.save(item);
}
@GetMapping("/items")
public List<Item> getAllItems() {
return repository.findAll();
}
@GetMapping("/items/{id}")
public Item getItemById(@PathVariable Long id) {
return repository.findById(id).orElse(null);
}
@PutMapping("/items/{id}")
public Item update(@PathVariable Long id, @RequestBody Item updatedItem) {
Item existingItem = repository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Item not found"));
existingItem.setName(updatedItem.getName());
existingItem.setPrice(updatedItem.getPrice());
return repository.save(existingItem);
}
@DeleteMapping("/items/{id}")
public ResponseEntity<?> delete(@PathVariable Long id) {
Item item = repository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Item not found"));
repository.delete(item);
return ResponseEntity.ok().build();
}
}
```
阅读全文
相关推荐
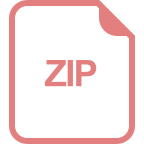
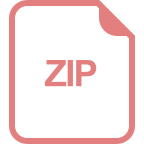
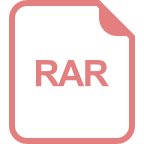
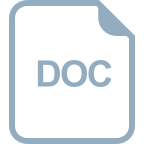
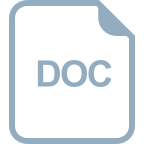






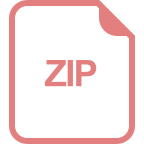
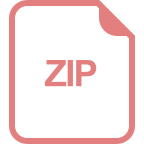
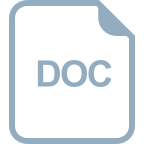
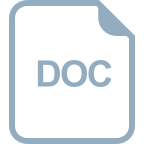
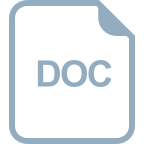

