写出计算一棵二叉树深度的算法(用二叉链存储结构),输出树的字符串表示形式,和其深度。
时间: 2024-12-12 08:25:50 浏览: 2
如果你使用的是二叉链表(即每个节点包含指向左右孩子的指针,而不是嵌套的Node对象),那么可以修改一下上面的递归算法。以下是用链表存储结构的Java代码示例:
```java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
}
}
public class BinaryTree {
public int maxDepth(TreeNode node) {
if (node == null) {
return 0;
} else {
int leftDepth = maxDepth(node.left);
int rightDepth = maxDepth(node.right);
// 更新树的深度并输出
System.out.println("TreeNode with value " + node.val + " has a depth of " + (Math.max(leftDepth, rightDepth) + 1));
return Math.max(leftDepth, rightDepth) + 1;
}
}
// 输出树的字符串表示形式
public String treeToString(TreeNode node, String prefix) {
if (node == null) {
return "";
}
String leftString = treeToString(node.left, prefix + "(" + node.val + ")");
String rightString = treeToString(node.right, prefix + "(" + node.val + ")");
return leftString + (rightString.isEmpty() ? "" : "\n" + prefix) + rightString;
}
}
// 示例用法
public static void main(String[] args) {
TreeNode root = new TreeNode(1);
// ...继续构建二叉树
BinaryTree bt = new BinaryTree();
bt.maxDepth(root); // 计算深度
System.out.println(bt.treeToString(root, ""));
}
```
在这个版本中,`treeToString`函数用于将二叉树转换成字符串,它会递归地遍历整个树,并添加适当的缩进。
阅读全文
相关推荐



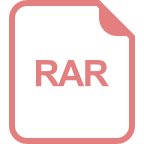
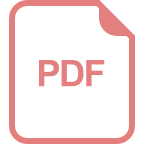
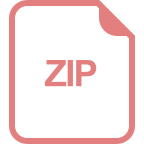
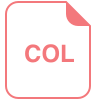
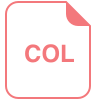
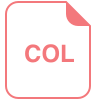
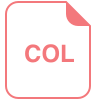
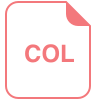
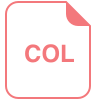
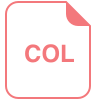
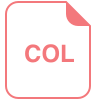
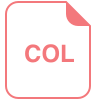
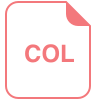
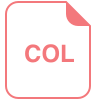
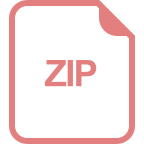
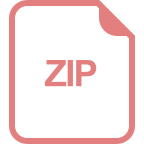