用eclipse写出计算一棵二叉树深度的算法(用二叉链存储结构),输出树的字符串表示形式,和其深度。
时间: 2024-12-12 19:28:07 浏览: 3
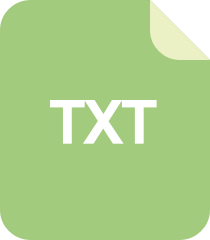
01_Java版数据结构与算法 02_算法_直通BAT算法精讲
在Eclipse中编写一个递归算法来计算二叉树的深度,我们可以使用二叉链存储结构。这里是一个基本的Java示例:
```java
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
// 构造函数
public TreeNode(int val) {
this.val = val;
left = null;
right = null;
}
}
public class BinaryTreeDepth {
private static int depth;
public static void calculateDepth(TreeNode root, String prefix) {
if (root == null) {
return;
}
// 计算当前节点的深度并更新全局深度
depth = Math.max(depth, prefix.length());
// 递归遍历左右子树
calculateDepth(root.left, prefix + "L->");
calculateDepth(root.right, prefix + "R->");
}
public static void main(String[] args) {
// 创建一个二叉树实例(这里仅作示例,实际应用需要根据具体树结构填充)
TreeNode tree = new TreeNode(1);
tree.left = new TreeNode(2);
tree.right = new TreeNode(3);
// ...继续添加子节点
// 初始化深度为0
depth = 0;
// 计算树的深度
calculateDepth(tree, "");
System.out.println("Tree as string: " + printInorder(tree)); // 打印树的字符串表示(例如: [1,L->[2],R->[3]])
System.out.println("Depth of the tree: " + depth);
}
// 中序遍历用于打印树的字符串表示
private static String printInorder(TreeNode node) {
StringBuilder sb = new StringBuilder();
if (node != null) {
sb.append(node.val).append("[");
sb.append(printInorder(node.left)).append(", ");
sb.append(printInorder(node.right)).append("]");
}
return sb.toString();
}
}
```
在这个例子中,`calculateDepth`函数通过递归遍历二叉树,并记录最长路径长度作为树的深度。`printInorder`方法则用于生成树的中序遍历字符串表示。
阅读全文
相关推荐
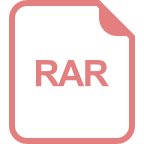
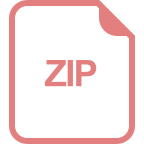

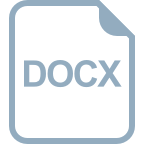
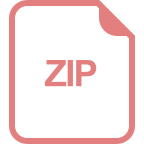
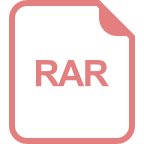
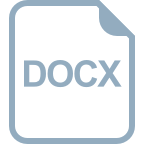
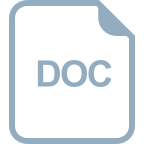
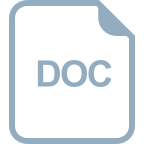
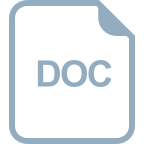
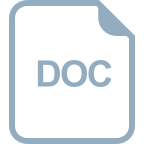
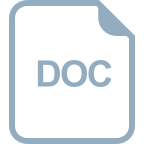
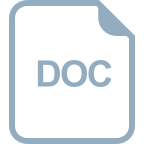
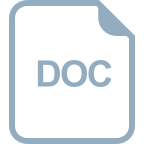
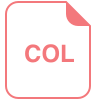
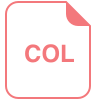
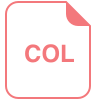
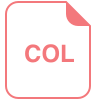
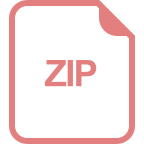