python代码测试GPU单精度算力
时间: 2024-09-28 22:00:48 浏览: 23
在Python中,你可以使用第三方库如`tensorflow`、`pytorch`或者`cupy`来测试GPU的单精度浮点运算性能。这里以`tensorflow`为例:
首先,确保已经安装了`tensorflow`,如果没有,可以使用pip安装:
```bash
pip install tensorflow
```
然后,你可以编写一段简单的代码来测量GPU上单精度(float32)运算的性能。例如,计算矩阵乘法就是一个常见的测试操作:
```python
import tensorflow as tf
# 创建两个随机生成的大张量
x = tf.random.normal(shape=(1000, 1000), dtype=tf.float32)
y = tf.random.normal(shape=(1000, 1000), dtype=tf.float32)
# 定义GPU加速的矩阵乘法函数
@tf.function(device='GPU')
def matmul_gpu(x, y):
return tf.matmul(x, y)
# 开始计时
start_time = time.time()
result_gpu = matmul_gpu(x, y)
end_time = time.time()
# 计算并打印单精度浮点运算时间
gpu_time = end_time - start_time
print(f"GPU单精度矩阵乘法的时间: {gpu_time}秒")
相关问题
pythonGPU单精度算力测试代码
在Python中,特别是在使用NVIDIA的cuPy库进行GPU计算时,我们可以编写一些简单的代码来测试单精度(float32)浮点数的算力。以下是使用cuPy的一个例子,它包含了一个基本的单精度矩阵乘法操作:
```python
import cupy as cp
# 定义GPU上的单精度数组
A = cp.random.rand(1024, 1024).astype(cp.float32)
B = cp.random.rand(1024, 1024).astype(cp.float32)
# 使用cupy.dot函数执行矩阵乘法(浮点32)
start_time = cp.cuda.Event()
end_time = cp.cuda.Event()
start_time.record()
result = cp.dot(A, B) # 这里就是单精度运算
end_time.record()
# 等待所有事件完成
cp.cuda.Stream.null.synchronize()
# 计算时间差
time_diff = end_time.time_since(start_time)
# 每秒浮点运算次数 (GFLOPS)
gflops = (result.size * 2) / time_diff / 1e9 # 因为每次乘法涉及两个单精度浮点数
print(f"单精度矩阵乘法在GPU上的运行时间为 {time_diff:.3f} 秒,单精度算力为大约 {gflops:.3f} GFLOPS")
```
这个代码片段首先创建了两个随机的1024x1024大小的单精度GPU数组,然后测量了矩阵乘法的时间,最后计算出每秒的浮点运算次数(GFLOPS)。请注意,实际性能可能会受到硬件限制和系统负载的影响。
pythonGPU双精度算力测试代码
在Python中,如果你想要测试GPU的双精度浮点数(通常指单精度为float64)计算能力,你可以利用深度学习库如PyTorch或TensorFlow提供的功能。下面是一个简单的例子,使用PyTorch:
```python
import torch
# 检查可用的GPU设备及其CUDA版本
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Running on {device}: CUDA version {torch.version.cuda}")
# 创建一个大的张量并将其移动到GPU上
large_tensor = torch.randn(1024, 1024).double().to(device)
start_time = torch.cuda.Event(enable_timing=True)
end_time = torch.cuda.Event(enable_timing=True)
# 执行一次双精度浮点运算,例如矩阵乘法
with torch.no_grad():
start_time.record()
result = torch.matmul(large_tensor, large_tensor.t())
end_time.record()
# 等待所有事件完成计时
torch.cuda.synchronize()
# 计算时间
time_taken = start_time.elapsed_time(end_time) / 1e3
print(f"Time taken for a double precision matrix multiplication: {time_taken:.6f} ms")
相关推荐
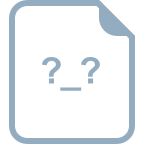
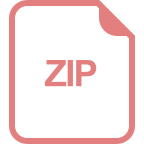
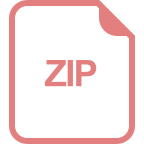
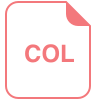
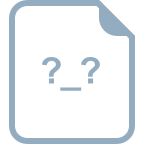
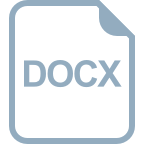
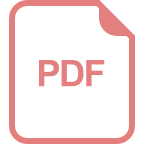
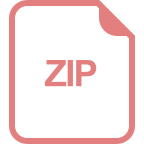
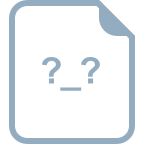
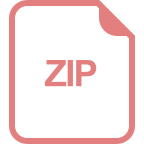
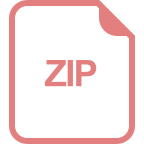
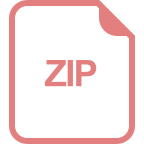
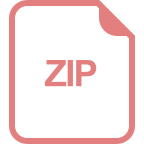
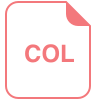
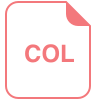
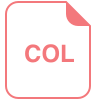
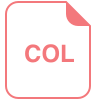