c++ image 转 nv21 代码函数
时间: 2024-12-16 21:21:49 浏览: 5
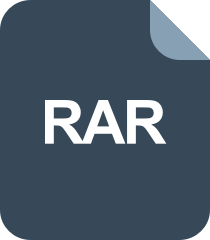
Java转C++代码工具 J2C

在C++中,将图像从常见的格式转换为NV21格式通常涉及到第三方库的帮助,因为NV21是一种视频编码格式,特别用于YUV420sp(也称为I420),常见于摄像头和H.264编码。以下是一个简化的示例,使用开源库如FFmpeg来进行这样的转换:
```cpp
#include <opencv2/opencv.hpp>
#include <ffmpeg.h>
void imageToNV21(const cv::Mat& inputImage, uint8_t* outputNV21) {
// FFmpeg需要一个文件流对象,这里我们暂时忽略输入流
AVFormatContext* formatCtx = nullptr;
AVCodecContext* codecCtx = nullptr;
AVFrame* frame = nullptr;
// 初始化FFmpeg
av_register_all();
if (avcodec_find_decoder(AV_CODEC_ID_H264) == nullptr) {
std::cerr << "Error: H264 decoder not found." << std::endl;
return;
}
// 创建解码器上下文
if (!avcodec_alloc_context3(&codecCtx)) {
std::cerr << "Error: Failed to allocate codec context." << std::endl;
return;
}
codecCtx->codec_id = AV_CODEC_ID_H264;
codecCtx->width = inputImage.cols;
codecCtx->height = inputImage.rows;
codecCtx->pix_fmt = AV_PIX_FMT_YUV420P; // 模拟H264格式
// 打开解码器并初始化帧
if (!avcodec_open2(codecCtx, nullptr, nullptr)) {
std::cerr << "Error: Failed to open codec." << std::endl;
return;
}
frame = av_frame_alloc();
// 遍历图像的每一行
for (int y = 0; y < codecCtx->height; ++y) {
// ...假设inputImage是逐行存储的BGR数据
// 将每一行的数据转换为NV21格式
for (int x = 0; x < codecCtx->width / 2; ++x) {
int idx_yuv = ((y * codecCtx->width + x) * 3); // YUV的索引计算
int idx_nv21_y = idx_yuv;
int idx_nv21_u = idx_yuv + 1; // U分量
int idx_nv21_v = idx_yuv + 2; // V分量
// 这里仅做简单的转换,实际应用需处理颜色空间转换
outputNV21[idx_nv21_y] = inputImage.at<cv::Vec3b>(y, x * 2).val[0];
outputNV21[idx_nv21_u] = (inputImage.at<cv::Vec3b>(y, x * 2 + 1).val[1] + inputImage.at<cv::Vec3b>(y, x * 2 + 2).val[1]) / 2;
outputNV21[idx_nv21_v] = inputImage.at<cv::Vec3b>(y, x * 2 + 1).val[0]; // U和V通道通常是相同的
}
}
// 结束后记得释放资源
av_frame_unref(frame);
avcodec_close(codecCtx);
avformat_free_context(formatCtx);
// 注意这只是一个基础示例,实际场景中你需要更完整的错误处理和内存管理
}
阅读全文
相关推荐
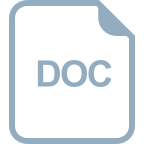
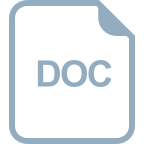
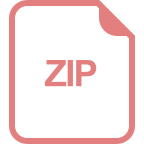
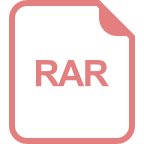
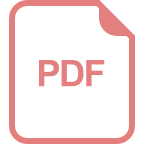
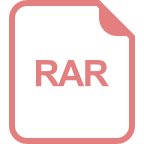
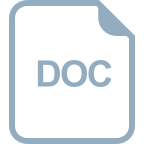




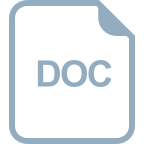
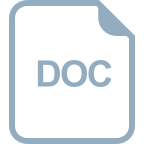
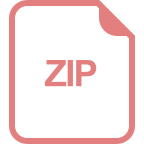
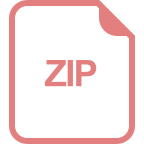