C#多个不同类型设备串口通讯程序示例
时间: 2024-10-08 15:19:08 浏览: 44
在C#中,通过SerialPort类可以实现多个不同类型的设备之间的串口通信。下面是一个简单的示例,假设我们有两个设备,一个使用COM1端口,另一个使用COM2端口:
```csharp
using System.IO.Ports;
// 定义一个设备接口
public interface IDevice
{
void Send(string data);
string Receive();
}
// COM1设备实现接口
class DeviceCom1 : IDevice
{
private SerialPort _serialPort;
public DeviceCom1()
{
_serialPort = new SerialPort("COM1", 9600); // 设置波特率和数据位
_serialPort.Open();
}
public void Send(string data)
{
_serialPort.WriteLine(data);
}
public string Receive()
{
return _serialPort.ReadLine();
}
}
// COM2设备实现接口
class DeviceCom2 : IDevice
{
private SerialPort _serialPort;
public DeviceCom2()
{
_serialPort = new SerialPort("COM2", 9600);
_serialPort.Open();
}
public void Send(string data)
{
_serialPort.WriteLine(data);
}
public string Receive()
{
return _serialPort.ReadLine();
}
}
// 主程序
class Program
{
static void Main(string[] args)
{
IDevice device1 = new DeviceCom1();
IDevice device2 = new DeviceCom2();
device1.Send("Hello, COM1!"); // 发送消息到COM1
Console.WriteLine(device2.Receive()); // 从COM2接收
device2.Send("Hi, COM2!"); // 发送消息到COM2
Console.WriteLine(device1.Receive()); // 从COM1接收
}
}
```
阅读全文
相关推荐








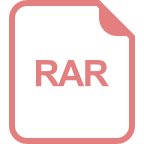


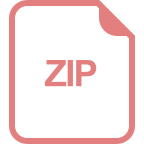


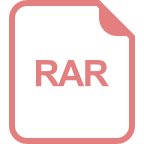



