输入一个十进制整数,倒序输出对应的二进制数。不要用数组和print_binary函数
时间: 2024-09-15 18:15:26 浏览: 71
当然,你可以使用位运算和循环的方式来实现这个功能。以下是一个简单的C语言程序,它接受一个十进制整数作为输入,然后逐位计算并打印出对应的二进制数:
```c
#include <stdio.h>
// Function to convert decimal to binary without using arrays or print_binary function
void decimalToBinary(int num) {
if (num == 0)
printf("0");
else {
while (num != 0) {
// Get the last bit and shift all bits to the right
int remainder = num & 1;
printf("%d", remainder);
// Shift the number to remove the last bit
num = num >> 1;
}
}
}
int main() {
int decimalInput;
printf("Enter a decimal integer: ");
scanf("%d", &decimalInput);
// Call the function to print the binary representation
decimalToBinary(decimalInput);
return 0;
}
```
在这个程序中,`& 1` 操作符用于获取数字的最低有效位(bit),`>> 1` 则右移所有的位,去掉最低位,然后重复这个过程直到数字变为0。
阅读全文
相关推荐

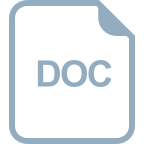
















