怎么用c语言打开四个文本文档读取数据后统计所有英文单词数量
时间: 2024-12-18 13:19:05 浏览: 17
在C语言中,要完成这个任务,你需要分几个步骤:
1. **包括头文件**:首先,需要包含一些基本的头文件,如`stdio.h`用于输入输出操作,`string.h`用于处理字符串。
2. **函数声明**:声明一个用于读取文件并统计英文单词数量的函数。
```c
#include <stdio.h>
#include <ctype.h> // 包含标准库函数isalpha()来判断字符是否为字母
// 定义统计单词数量的函数
int count_words(char* filename);
```
3. **主函数**:在主函数main()中,创建四个文件指针,并循环打开每个文档,然后调用`count_words()`函数。
```c
int main() {
char filenames[] = {"file1.txt", "file2.txt", "file3.txt", "file4.txt"};
int num_files = sizeof(filenames) / sizeof(filenames[0]);
for (int i = 0; i < num_files; i++) {
FILE* file = fopen(filenames[i], "r");
if (file == NULL) {
printf("Error opening file %s\n", filenames[i]);
continue;
}
int word_count = count_words(filenames[i]); // 调用计数函数
fclose(file); // 关闭文件
// 打印或处理单词数量
printf("File %s has %d English words.\n", filenames[i], word_count);
}
return 0;
}
```
4. **`count_words` 函数**:此函数逐行读取文件内容,检查每个字符是否为字母,并统计非空格的连续字母作为单词。
```c
int count_words(char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
int word_count = 0;
char buffer[1024]; // 预设缓冲区大小
while (fgets(buffer, sizeof(buffer), file)) {
size_t len = strlen(buffer);
bool is_word = true;
for (size_t i = 0; i < len; i++) {
if (!isalpha(buffer[i]) && buffer[i] != '_') { // 不考虑特殊字符和下划线
is_word = false;
break;
} else if (isspace(buffer[i])) { // 空格结束单词
is_word = false;
}
}
if (is_word) {
word_count++;
}
}
fclose(file);
return word_count;
}
```
阅读全文
相关推荐
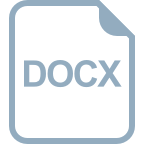
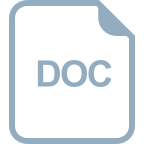
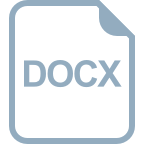

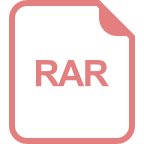
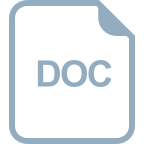
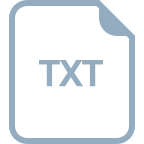
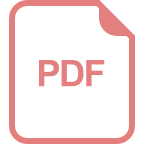
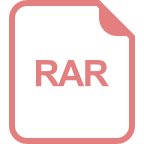
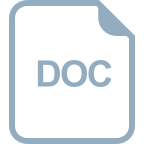
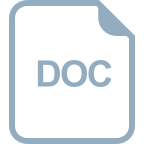
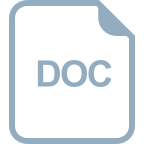
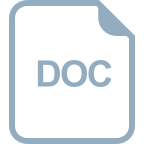
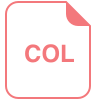
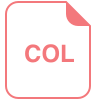
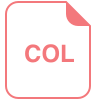
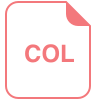