怎么用c语言打开四个文本文档读取数据,并统计英文单词数量
时间: 2024-12-18 08:19:02 浏览: 11
在C语言中,为了打开并读取四个文本文档并统计其中的英文单词数量,你可以按照以下步骤操作:
1. **包含头文件**:
首先,需要包含`stdio.h`以处理输入输出,`stdlib.h`以获取动态内存,以及`string.h`用于字符串处理。
2. **函数声明**:
定义一个函数来逐行读取文本内容,如`count_words`。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
size_t count_words(char* filename);
```
3. **读取文件内容**:
使用`fopen`打开每个文件,然后循环读取每一行,使用`fgets`获取行内容,再分割成单词。
```c
size_t count_words(char* filename) {
FILE* file = fopen(filename, "r");
if (!file) {
perror("Error opening file");
return 0;
}
char line[1024]; // 一个足够大的缓冲区
size_t word_count = 0;
while (fgets(line, sizeof(line), file)) {
size_t len = strlen(line);
// 将末尾的换行符移除
if (line[len - 1] == '\n') {
line[len - 1] = '\0';
}
// 拆分单词并计数
char* token = strtok(line, " ");
while (token != NULL) {
++word_count;
token = strtok(NULL, " ");
}
}
fclose(file); // 关闭文件
return word_count;
}
```
4. **遍历并统计所有文档**:
创建一个数组存储文件名,遍历数组并调用上述函数计算每个文档的单词数量。
```c
int main() {
const char* filenames[] = {"file1.txt", "file2.txt", "file3.txt", "file4.txt"};
int num_files = sizeof(filenames) / sizeof(filenames[0]);
for (int i = 0; i < num_files; ++i) {
size_t words_in_file = count_words(filenames[i]);
printf("File %s contains %zu English words.\n", filenames[i], words_in_file);
}
return 0;
}
```
阅读全文
相关推荐
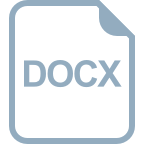
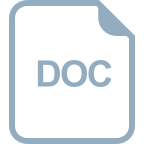
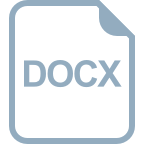

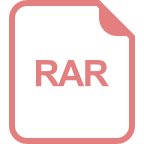
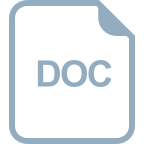
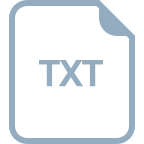
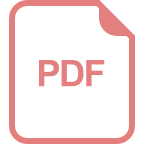
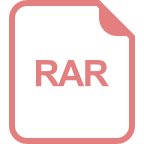
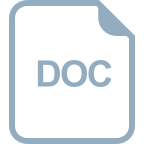
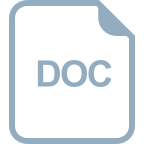
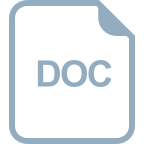
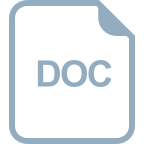
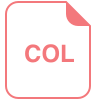
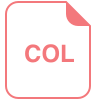
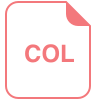
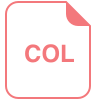
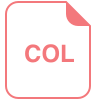
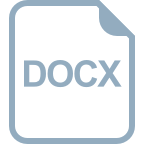