std::vector<int> 累加
时间: 2024-08-26 08:00:37 浏览: 63
`std::vector<int>` 是 C++ 标准库中的动态数组,可以存储一组整数。如果你想累加这个向量中的所有元素,你可以直接遍历整个向量,并将每个元素相加。这里有一个简单的示例:
```cpp
#include <iostream>
#include <vector>
int sum(std::vector<int>& vec) {
int sum = 0; // 初始化累加器为0
for (const auto& element : vec) {
sum += element; // 累加当前元素到sum
}
return sum;
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int total = sum(numbers);
std::cout << "The sum of the vector elements is: " << total << std::endl;
return 0;
}
```
上述代码中,`sum` 函数接收一个 `std::vector<int>` 的引用,然后通过一个范围-based for 循环,逐个累加元素。最后返回总和。
相关问题
vector<vector<int>> 怎样知道二维数组的行数和列数
`vector<vector<int>>` 表示的是一个动态大小的二维整数向量,也称为动态数组或矩阵。要获取这个二维数组(矩阵)的行数和列数,你可以通过迭代每个内部的 `vector<int>` 来计数。
以下是一个简单的步骤:
1. 初始化两个变量,如 `rows` 和 `cols`,分别用于存储行数和列数,初始值设为0。
2. 使用嵌套循环遍历矩阵中的元素。外层循环遍历每一行,内层循环遍历每一列。
3. 对于每行,访问完所有列后,将 `rows` 自增 1。
4. 对于每个列,由于我们是在处理矩阵中的一个 `vector`,所以列数即为每个 `vector` 的长度,因此不需要额外的循环,直接累加到 `cols` 即可。
如果你想要一个更通用的方法,可以使用 C++ 的标准库函数,例如 `<vector>` 中的 `size()` 函数,但需要稍微复杂一些,因为需要分别计算内、外层 `vector` 的尺寸。下面是一个代码片段演示如何做到这一点:
```cpp
std::vector<std::vector<int>> matrix;
// ...填充矩阵...
int rows = matrix.size(); // 获取行数
if (rows > 0) {
int cols = matrix[0].size(); // 获取列数,假设所有行的列数都一样
// ...现在你知道行数和列数了...
}
```
请你解析下列代码#include <iostream>#include <vector>#include <cstdlib>#include <ctime>#include <chrono>#include <thread>class Grid {public: Grid(int width, int height) : width_(width), height_(height) { grid_.resize(width_ * height_); for (int i = 0; i < grid_.size(); ++i) { grid_[i] = rand() % 2; } } void update() { std::vector<int> new_grid(grid_.size()); for (int i = 0; i < height_; ++i) { for (int j = 0; j < width_; ++j) { int count = live_neighbors(j, i); int index = i * width_ + j; if (count == 3 || (count == 2 && grid_[index])) { new_grid[index] = 1; } else { new_grid[index] = 0; } } } grid_ = new_grid; } void print() { for (int i = 0; i < height_; ++i) { for (int j = 0; j < width_; ++j) { int index = i * width_ + j; if (grid_[index]) { std::cout << "#"; } else { std::cout << " "; } } std::cout << std::endl; } }private: int live_neighbors(int x, int y) { int count = 0; for (int j = -1; j <= 1; ++j) { for (int i = -1; i <= 1; ++i) { int col = (x + i + width_) % width_; int row = (y + j + height_) % height_; int index = row * width_ + col; count += grid_[index]; } } count -= grid_[y * width_ + x]; return count; } int width_; int height_; std::vector<int> grid_;};int main() { srand(time(nullptr)); int width, height; std::cout << "Enter grid width: "; std::cin >> width; std::cout << "Enter grid height: "; std::cin >> height; Grid grid(width, height); while (true) { grid.print(); std::this_thread::sleep_for(std::chrono::milliseconds(500)); grid.update(); } return 0;}
这段代码实现了一个经典的“生命游戏”(Game of Life),它是一种细胞自动机,由英国数学家约翰·康威于1970年发明。
首先,在头文件中包含了一些标准库和自定义的类 Grid。
Grid 类有三个公有成员函数:构造函数 Grid(int width, int height)、更新函数 update() 和打印函数 print()。Grid 类还有三个私有成员变量:宽度 width_,高度 height_,和一个一维向量 grid_,用于存储每个细胞的状态(0 或 1)。
在主函数 main() 中,首先使用 srand(time(nullptr)) 函数初始化随机数种子,然后通过 std::cin 输入细胞网格的宽度和高度,创建一个 Grid 对象 grid,并在一个无限循环中,不断打印细胞网格的状态,暂停 500 毫秒后更新细胞状态。
在 Grid 类的构造函数中,先将 grid_ 向量的大小设置为 width_ * height_,然后使用 rand() 函数随机设置每个细胞的状态,0 表示死亡,1 表示存活。
在 Grid 类的更新函数 update() 中,首先创建一个新的向量 new_grid,用于存储下一代细胞的状态。然后遍历每个细胞,计算它周围存活的细胞数量,根据生命游戏的规则判断它下一代的状态,并将结果存储在 new_grid 中。最后将 grid_ 向量更新为 new_grid 向量。
在 Grid 类的打印函数 print() 中,遍历每个细胞,如果它存活,则输出 "#",否则输出空格。每行细胞之间输出换行符。
在 Grid 类的私有成员函数 live_neighbors(int x, int y) 中,计算细胞 (x, y) 周围存活的细胞数量。遍历以 (x, y) 为中心的 9 个细胞,并累加存活的细胞数量。最后减去细胞 (x, y) 自己的状态,得到周围存活细胞的数量。
总体来说,这段代码实现了生命游戏的基本功能,通过控制台打印出了每一代细胞的状态,并且每隔 0.5 秒自动更新一次。
相关推荐
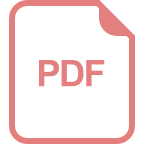
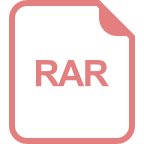
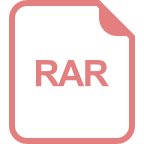












