如何在Java JDK 8中使用内置的HttpURLConnection类实现HTTP请求?能否提供一个详细的示例代码片段?
时间: 2024-10-23 08:18:28 浏览: 16
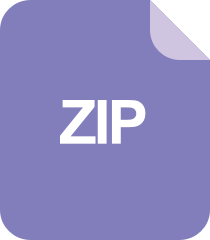
jdk9全版本 java9 Jdk9.zip
在Java JDK 8中,我们可以使用`java.net.HttpURLConnection`类来发送HTTP请求,这是一个低级别的网络连接API,适合处理基本的GET、POST等请求。下面是一个简单的示例代码片段:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpRequestExample {
public static void main(String[] args) throws Exception {
// 创建URL对象,指定你要访问的服务器地址和资源路径
URL url = new URL("http://example.com/api/resource");
// 创建HttpURLConnection实例
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法(GET、POST等)
connection.setRequestMethod("GET"); // 或者 "POST" if you have data to send
// 设置请求头,如若需要
connection.setRequestProperty("User-Agent", "Mozilla/5.0");
connection.setRequestProperty("Content-Type", "application/json"); // 如果是POST请求并且有JSON数据
// 打开连接并获取响应码
int responseCode = connection.getResponseCode();
System.out.println("Response Code : " + responseCode);
// 检查请求是否成功,通常2xx表示成功
if (responseCode >= 200 && responseCode < 300) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuffer content = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
// 输出响应内容
System.out.println(content.toString());
} else {
// 错误处理,比如404 Not Found,500 Internal Server Error等
System.out.println("Failed : HTTP error code : " + responseCode);
}
// 关闭连接
connection.disconnect();
}
}
```
在这个例子中,我们首先创建了一个URL对象,然后通过`openConnection()`方法打开到该URL的连接,并设置请求方法。对于GET请求,通常不需要设置额外的数据,而对于POST或其他需要数据发送的请求,还需要设置请求体。最后,读取响应并关闭连接。
阅读全文
相关推荐
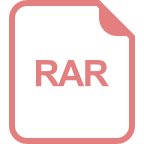
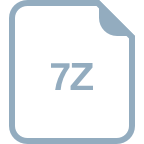
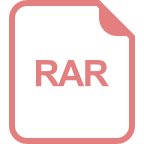
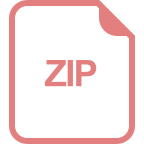
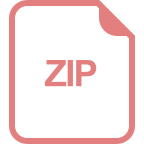
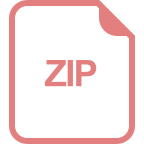
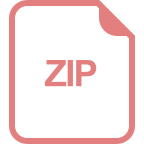
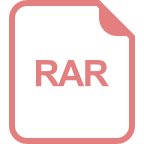
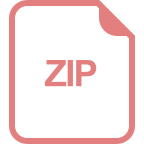
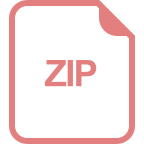
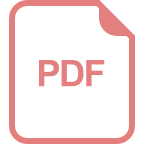
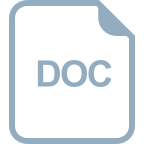
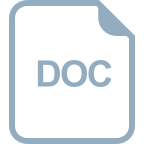
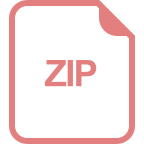
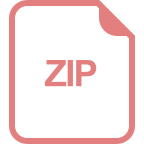