将笛卡尔坐标系上的点定义为一个类Point,该类要求提供求得坐标系上两点间距离的功能、获取和设置坐标的功能、获取极坐标的功能,以及完成对已创建的Point类对象进行个数统计的功能。设计测试Point类的应用程序主类,用中文测试并显示输出提供所有功能的结果。
时间: 2024-10-21 22:02:42 浏览: 48
在Python中,我们可以设计一个名为`Point`的类,用于表示二维笛卡尔坐标系上的点。以下是这个类的设计:
```python
import math
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
# 获取或设置坐标
@property
def coordinates(self):
return self.x, self.y
@coordinates.setter
def coordinates(self, value):
self.x, self.y = value
# 计算两点之间的距离
def distance_to(self, other_point):
dx = self.x - other_point.x
dy = self.y - other_point.y
return math.sqrt(dx**2 + dy**2)
# 转换为极坐标
def to_polar(self):
radius = math.sqrt(self.x**2 + self.y**2)
theta = math.atan2(self.y, self.x)
return radius, theta
# 点的数量统计(假设我们有一个point_list)
@classmethod
def count_points(cls, point_list):
return len(point_list)
# 测试Point类
def test_point_class():
p1 = Point(3, 4)
print(f"点 {p1.coordinates} 到原点的距离为: {p1.distance_to(Point())}")
p2 = Point(-2, 5)
print(f"从 {p1.coordinates} 到 {p2.coordinates} 的距离为: {p1.distance_to(p2)}")
p1.coordinates = (-1, 1)
print(f"更新坐标后,{p1.coordinates} 的极坐标是: {p1.to_polar()}")
points = [Point(1, 1), Point(2, 2), Point(0, 0)]
print(f"点列表中有 {Point.count_points(points)} 个点")
if __name__ == "__main__":
test_point_class()
```
在这个示例中,`test_point_class()`函数包含了对`Point`类各项功能的测试,包括距离计算、坐标设置和转换以及点的数量统计。
运行上述代码会得到相应的输出结果,展示了每个功能的执行情况。
阅读全文
相关推荐
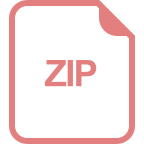
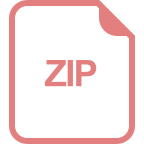

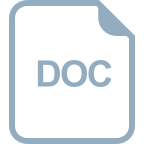
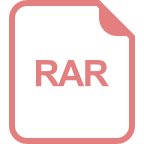
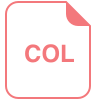
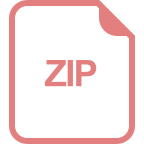
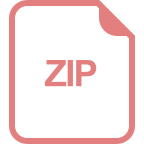
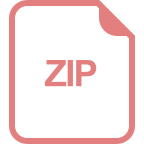
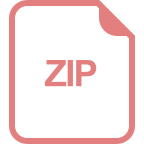
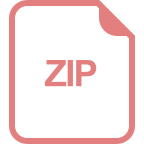
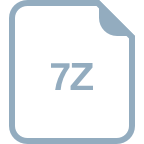
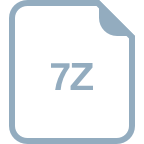
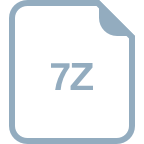
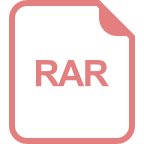
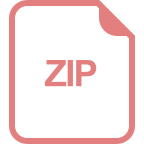
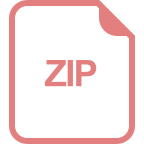