用栈实现非递归迷宫问题算法,C语言,从1,1到m,n,自己输入迷宫,与DFS有关,打印完整代码
时间: 2024-12-15 07:16:46 浏览: 24
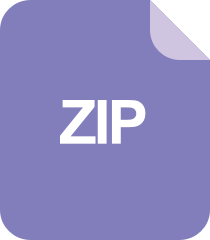
c语言实现迷宫问题:创建迷宫,自动寻路
在C语言中,我们可以使用栈数据结构和深度优先搜索(DFS)策略来解决迷宫问题,这里假设迷宫是一个二维数组,0表示通路,1表示墙壁。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 5 // 迷宫行数
#define COLS 5 // 迷宫列数
typedef struct {
int row;
int col;
} Cell;
// 检查当前位置是否合法
int is_valid(Cell cell, int maze[ROWS][COLS]) {
return cell.row >= 0 && cell.row < ROWS && cell.col >= 0 && cell.col < COLS && maze[cell.row][cell.col] == 0;
}
// 栈操作函数
void push(Stack *stack, Cell cell) {
stack->cells[stack->top++] = cell;
}
Cell pop(Stack *stack) {
return stack->cells[--stack->top];
}
// 非递归DFS
void maze_solver(int maze[][COLS], Stack *stack, Cell start) {
stack->top = 0;
push(stack, start);
while (stack->top > 0) {
Cell current = pop(stack);
printf("(%d,%d) ", current.row + 1, current.col + 1); // 打印路径
if (current.row + 1 < ROWS && maze[current.row + 1][current.col] == 0) { // 向下
maze[current.row + 1][current.col] = 1; // 标记已访问
push(stack, (Cell){current.row + 1, current.col});
}
else if (current.col + 1 < COLS && maze[current.row][current.col + 1] == 0) { // 右边
maze[current.row][current.col + 1] = 1;
push(stack, (Cell){current.row, current.col + 1});
}
else if (current.row - 1 >= 0 && maze[current.row - 1][current.col] == 0) { // 上面
maze[current.row - 1][current.col] = 1;
push(stack, (Cell){current.row - 1, current.col});
}
else if (current.col - 1 >= 0 && maze[current.row][current.col - 1] == 0) { // 左边
maze[current.row][current.col - 1] = 1;
push(stack, (Cell){current.row, current.col - 1});
}
}
}
int main() {
int maze[ROWS][COLS] = {
{0, 0, 0, 0, 1},
{0, 1, 0, 1, 1},
{0, 0, 0, 1, 1},
{1, 1, 1, 1, 1},
{0, 0, 0, 0, 0}
};
Stack stack;
stack.top = 0;
Cell start = {0, 0};
maze_solver(maze, &stack, start);
printf("\nPath not found.\n");
return 0;
}
阅读全文
相关推荐
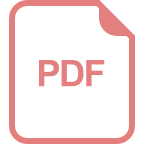
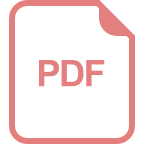

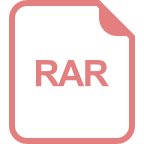
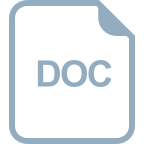

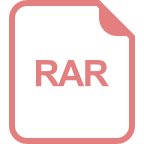
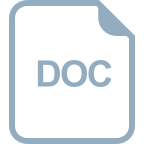
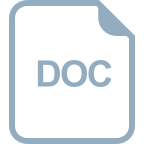
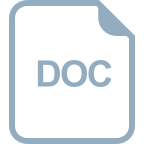
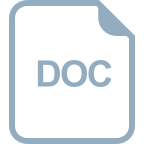
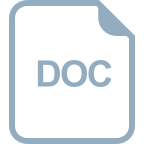





