大学Python习题 编写一个能搜索单词的程序
时间: 2024-09-30 15:15:05 浏览: 51
编写一个能搜索单词的大学Python程序,通常会涉及到用户输入、数据存储(如列表或字典)以及关键词匹配功能。下面是一个简单的示例,这个程序会在给定的一组字符串中查找用户指定的单词:
```python
def search_word():
# 创建一个包含单词的列表
word_list = ['apple', 'banana', 'cherry', 'date', 'elderberry']
# 获取用户输入的单词
user_input = input("请输入要查找的单词:")
# 检查用户输入是否为空
if not user_input:
print("请输入有效的单词")
else:
# 查找并打印出结果
if user_input in word_list:
print(f"'{user_input}' 在词汇列表中.")
else:
print(f"'{user_input}' 没有找到在词汇列表中.")
# 调用函数
search_word()
```
在这个程序中,你可以添加更多的功能,比如从文件中读取单词列表,或者支持模糊匹配等高级搜索。
相关问题
大学Python习题 编写一个能搜索单词的程序,函数名为def exist(board: List[List[str]], word: str)
在大学Python学习中,编写这样的单词搜索程序可以锻炼学生的列表操作、字符串处理和遍历理解能力。`exist` 函数的作用是在给定的二维字符网格 `board` 中查找是否存在指定的单词 `word`。这个函数通常需要采用宽度优先搜索(广度优先搜索)或深度优先搜索算法来逐行逐列地检查每个字符是否匹配,并保持追踪已访问的位置。
这是一个简单的示例实现:
```python
from typing import List
def is_valid(i, j, board):
# 检查索引是否越界并确保当前位置不是空格
return 0 <= i < len(board) and 0 <= j < len(board[0]) and board[i][j] != ' '
def exist(board: List[List[str]], word: str) -> bool:
rows, cols = len(board), len(board[0])
def dfs(i, j, current_word=()):
# 初始化当前路径为空串
if current_word == (word,):
return True
# 搜索上下左右四个方向
for dx, dy in [(0, -1), (-1, 0), (0, 1), (1, 0)]:
ni, nj = i + dx, j + dy
if is_valid(ni, nj) and board[ni][nj] == current_word[-1]:
new_word = current_word[:-1] + (board[ni][nj],)
if dfs(ni, nj, new_word):
return True
return False
# 从每个位置开始搜索
for i in range(rows):
for j in range(cols):
if board[i][j] == word[0]:
if dfs(i, j):
return True
# 如果都没有找到,返回False
return False
# 示例
board = [['A', 'B', 'C', 'E'], ['S', 'F', 'C', 'S'], ['A', 'D', 'E', 'E']]
word = "ABCCED"
print(exist(board, word)) # 输出:True
```
网安python练习题
以下是一些网安Python练习题的例子:
1. 编写一个程序,该程序接受一个字符串并计算其中大写字母和小写字母的数量。
```python
def count_upper_lower(s):
upper_count = 0
lower_count = 0
for c in s:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
return upper_count, lower_count
s = "Hello World"
upper_count, lower_count = count_upper_lower(s)
print("Number of uppercase letters:", upper_count) # 输出:2
print("Number of lowercase letters:", lower_count) # 输出:8
```
2. 编写一个程序,该程序接受一个字符串并将其中的元音字母替换为“*”。
```python
def replace_vowels(s):
vowels = "aeiouAEIOU"
for c in vowels:
s = s.replace(c, "*")
return s
s = "Hello World"
s = replace_vowels(s)
print(s) # 输出:H*ll* W*rld
```
3. 编写一个程序,该程序接受一个字符串并将其中的每个单词的首字母大写。
```python
def capitalize_words(s):
words = s.split()
capitalized_words = [word.capitalize() for word in words]
return " ".join(capitalized_words)
s = "hello world"
s = capitalize_words(s)
print(s) # 输出:Hello World
```
阅读全文
相关推荐
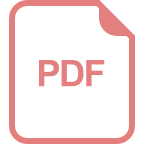
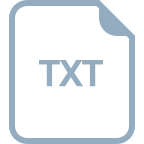
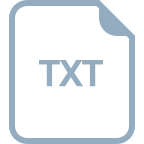
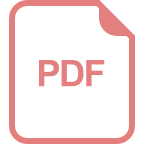
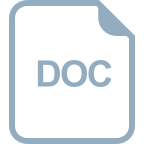










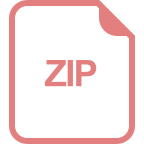