360度全景拼接代码
时间: 2023-12-20 09:30:08 浏览: 127
根据提供的引用内容,360度全景拼接的基本原理是透视变换。下面是一个使用OpenCV库实现360度全景拼接的Python代码示例:
```python
import cv2
import numpy as np
# 读取图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
img3 = cv2.imread('image3.jpg')
img4 = cv2.imread('image4.jpg')
# 设置拼接图像的大小和输出图像的大小
width, height = 960, 480
size = (width, height)
# 设置透视变换的参数
fov = 180
theta = fov / 2 * np.pi / 180
f = width / 2 / np.tan(theta / 2)
# 计算透视变换矩阵
K = np.array([[f, 0, width / 2], [0, f, height / 2], [0, 0, 1]])
R1 = np.array([[1, 0, 0], [0, np.cos(np.pi / 2), -np.sin(np.pi / 2)], [0, np.sin(np.pi / 2), np.cos(np.pi / 2)]])
R2 = np.array([[np.cos(np.pi), 0, -np.sin(np.pi)], [0, 1, 0], [np.sin(np.pi), 0, np.cos(np.pi)]])
R3 = np.array([[1, 0, 0], [0, np.cos(-np.pi / 2), -np.sin(-np.pi / 2)], [0, np.sin(-np.pi / 2), np.cos(-np.pi / 2)]])
R4 = np.array([[np.cos(np.pi / 2), 0, -np.sin(np.pi / 2)], [0, 1, 0], [np.sin(np.pi / 2), 0, np.cos(np.pi / 2)]])
P1 = K.dot(R1).dot(np.array([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 1, 0]])).dot(K)
P2 = K.dot(R2).dot(np.array([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 1, 0]])).dot(K)
P3 = K.dot(R3).dot(np.array([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 1, 0]])).dot(K)
P4 = K.dot(R4).dot(np.array([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 1, 0]])).dot(K)
# 进行透视变换
dst1 = cv2.warpPerspective(img1, P1, size)
dst2 = cv2.warpPerspective(img2, P2, size)
dst3 = cv2.warpPerspective(img3, P3, size)
dst4 = cv2.warpPerspective(img4, P4, size)
# 拼接图像
result = np.zeros((height * 2, width * 2, 3), dtype=np.uint8)
result[0:height, 0:width] = dst1
result[0:height, width:width * 2] = dst2
result[height:height * 2, 0:width] = dst3
result[height:height * 2, width:width * 2] = dst4
# 显示结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:上述代码仅为示例,实际使用时需要根据具体情况进行调整。
阅读全文
相关推荐
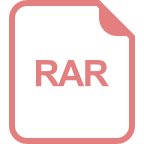
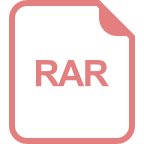
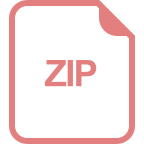
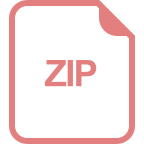
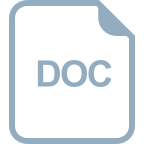
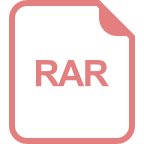
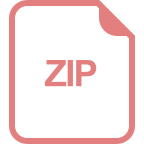
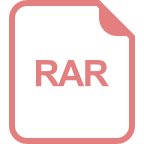
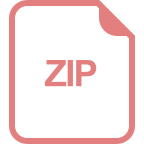
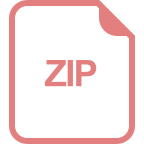
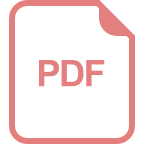
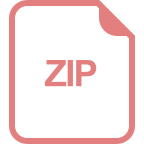
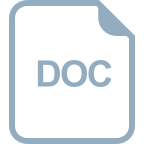


