c语言一句话单词频率统计
时间: 2024-11-15 15:13:31 浏览: 4
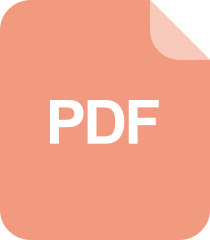
C语言统计一篇英文短文中单词的个数实例代码

C语言中,要统计一句话中每个单词的频率,可以采用以下步骤:
1. **预处理**: 将句子转换成小写并去除标点符号。
2. **分割单词**: 使用`strtok()`函数将句子分解成单个单词。
3. **存储频率**: 创建一个结构体或数组来存储每个单词及其出现次数,如键值对形式。
4. **遍历和计数**: 遍历每个单词,如果在存储结构中找到,则增加对应的计数;若未找到,则添加新条目并设为1。
5. **更新频率**: 每次遇到单词,都会检查并更新相应的计数。
下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 100
typedef struct {
char word[MAX_WORDS];
int count;
} WordCount;
WordCount word_counts[MAX_WORDS];
void count_words(char* sentence) {
char* token = strtok(sentence, " ,.;:\"\'\t\n");
while (token != NULL) {
strcpy(word_counts[token - sentence], token);
for (int i = 0; i < MAX_WORDS; ++i) {
if (strcmp(word_counts[i].word, token) == 0) {
word_counts[i].count++;
break;
}
}
token = strtok(NULL, " ,.;:\"\'\t\n");
}
}
int main() {
char sentence[] = "This is a sample sentence with words that will be counted.";
count_words(sentence);
// 输出结果
for (int i = 0; i < MAX_WORDS; ++i) {
if (word_counts[i].count > 0) {
printf("%s: %d\n", word_counts[i].word, word_counts[i].count);
}
}
return 0;
}
```
阅读全文
相关推荐
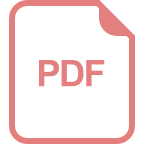
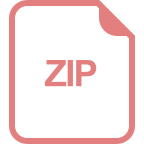
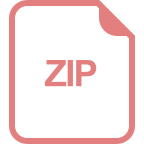
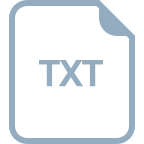
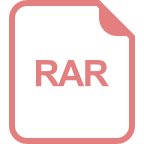
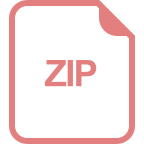
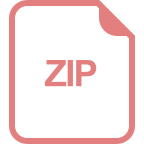
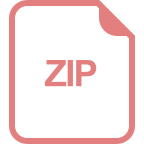
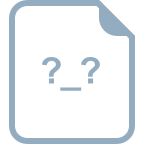
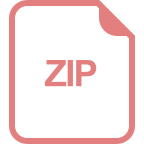