vba socket
时间: 2023-10-31 10:58:01 浏览: 217
VBA (Visual Basic for Applications) doesn't have built-in support for sockets. However, you can use external libraries or APIs to work with sockets in VBA. One popular choice is the Winsock API, which allows you to create socket connections and perform socket operations in VBA.
To use the Winsock API in VBA, you need to declare the necessary functions and structures from the Windows API. Here's an example of how you can establish a socket connection using the Winsock API in VBA:
```vba
Option Explicit
Private Declare Function WSAStartup Lib "wsock32.dll" (ByVal wVersionRequested As Integer, lpWSAData As WSADATA) As Integer
Private Declare Function socket Lib "wsock32.dll" (ByVal af As Integer, ByVal socktype As Integer, ByVal protocol As Integer) As Long
Private Declare Function connect Lib "wsock32.dll" (ByVal s As Long, addr As SOCKADDR, ByVal namelen As Long) As Long
Private Type WSADATA
wVersion As Integer
wHighVersion As Integer
szDescription(0 To 255) As Byte
szSystemStatus(0 To 128) As Byte
iMaxSockets As Integer
iMaxUdpDg As Integer
lpVendorInfo As Long
End Type
Private Type SOCKADDR
sin_family As Integer
sin_port As Integer
sin_addr As Long
sin_zero(0 To 7) As Byte
End Type
Sub ConnectToServer()
Dim wsaData As WSADATA
Dim clientSocket As Long
Dim serverAddress As SOCKADDR
' Initialize Winsock
If WSAStartup(32768 + 2, wsaData) <> 0 Then
MsgBox "Failed to initialize Winsock."
Exit Sub
End If
' Create socket
clientSocket = socket(2, 1, 0) ' AF_INET = 2, SOCK_STREAM = 1
' Set server address details
serverAddress.sin_family = 2 ' AF_INET
serverAddress.sin_port = htons(80) ' Port number (in this case, port 80)
serverAddress.sin_addr = inet_addr("192.168.0.1") ' Server IP address
' Connect to the server
If connect(clientSocket, serverAddress, LenB(serverAddress)) <> 0 Then
MsgBox "Failed to connect to the server."
Exit Sub
End If
' Socket connection successful
MsgBox "Connected to the server!"
' Close the socket
closesocket clientSocket
End Sub
```
Please note that this example code is for demonstration purposes only and may need modifications based on your specific requirements. Additionally, you may need to include additional error handling and cleanup routines.
Remember to reference the Winsock API (wsock32.dll) in your VBA project by going to "Tools" > "References" and selecting "Winsock Control" or "Microsoft Winsock Control".
I hope this helps you get started with sockets in VBA! Let me know if you have any further questions.
阅读全文
相关推荐
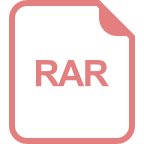
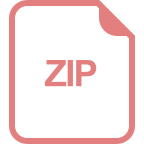
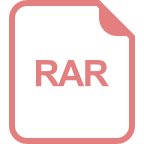
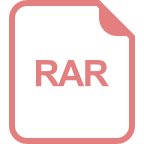
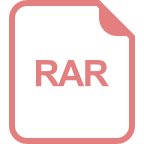
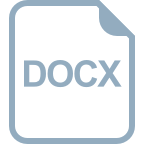
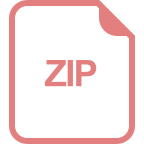
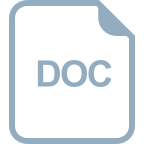




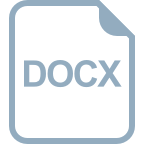