c++ 中std::thread 的用法
时间: 2024-12-07 09:13:31 浏览: 9
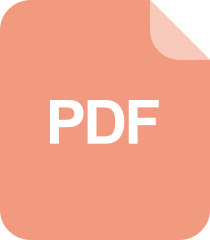
c++11中关于std::thread的join的详解
在C++中,`std::thread`是一个用于创建新线程并执行特定任务的库功能。它允许你编写并发程序,使得主线程和其他线程可以并行运行。以下是基本的`std::thread`用法:
1. 创建一个新的线程:
```cpp
#include <iostream>
#include <thread>
// 要在线程中执行的函数
void my_function() {
std::cout << "Hello from a separate thread!\n";
}
int main() {
// 创建新的线程并传入要执行的函数
std::thread worker_thread(my_function);
// 等待线程开始执行,然后继续主线程
worker_thread.detach(); // 如果不需要等待线程结束,可以使用detach()
// 主线程继续执行其他操作
std::cout << "Continuing in the main thread...\n";
return 0;
}
```
2. 使用lambda表达式:
```cpp
#include <iostream>
#include <thread>
int main() {
std::thread worker_thread([]() {
std::cout << "Hello from lambda function in a separate thread.\n";
});
worker_thread.join(); // 这里选择join()来等待线程完成
return 0;
}
```
3. 异步执行(如果不关心结果):
```cpp
#include <iostream>
#include <future>
int main() {
auto future = std::async(std::launch::async, []() {
std::cout << "Hello from an async task.\n";
});
// 不等待异步任务完成,main将继续执行
// future.get(); // 如果需要得到结果,可以在这里阻塞直到完成
std::cout << "Continuing in the main thread...\n";
return 0;
}
```
注意:
- `std::thread`对象一旦离开作用域,会自动销毁,释放线程资源。
- 使用`join()`方法可以让主线程等待线程执行完毕。
- `detach()`方法将线程从主进程分离,不会等待其结束,适合用于后台任务。
- 当在一个线程中抛出异常时,如果没有捕获,其他线程会收到终止信号(除非使用`std::async`结合`future`处理异常)。
阅读全文
相关推荐
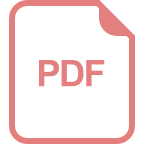
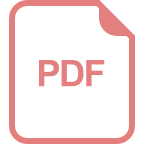




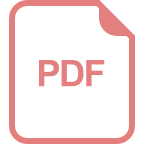
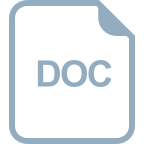
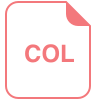
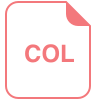
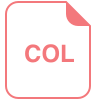
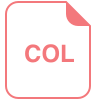
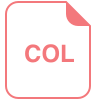
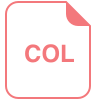
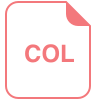
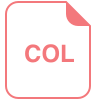

