netty sip 协议
时间: 2023-07-29 11:02:03 浏览: 473
Netty SIP协议是一种基于Netty框架的会话初始化协议。SIP代表会话初始化协议,它是一种基于文本的通信协议,用于在IP网络上建立、修改和终止多媒体会话。Netty是一个基于Java的异步事件驱动的网络应用编程框架,提供了高性能、可扩展的网络通信能力。
Netty SIP协议的设计目标是提供一个可靠、高速的会话初始化协议,以满足多媒体会话的需求。它基于Netty的事件驱动模型和异步IO,能够在网络通信中实现高性能和低延迟。
Netty SIP协议主要包含以下几个核心组件:
1. SIP消息格式:Netty SIP协议定义了SIP消息的格式,包括请求和响应的格式。这样可以方便地将SIP消息进行编解码,并在网络上进行传输。
2. SIP状态管理:Netty SIP协议通过状态管理来处理会话的各种状态。它能够跟踪会话的建立、修改和终止,并在需要时触发相应的事件。
3. 路由与转发:Netty SIP协议支持路由和转发功能,能够根据会话的相关信息将消息正确地转发到目标节点。这样可以构建一个分布式的会话系统。
4. 会话管理:Netty SIP协议提供了会话管理功能,能够管理会话的生命周期和相关的资源。它允许创建、销毁和管理会话,以及处理会话的各种事件。
总的来说,Netty SIP协议是一个基于Netty框架的高性能、可扩展的会话初始化协议。它能够实现多媒体会话的建立、修改、终止和管理,为网络通信提供了可靠和高效的解决方案。
相关问题
netty自定义协议demo
以下是一个简单的Netty自定义协议的示例代码,该协议由消息头和消息体组成,消息头包含消息的长度信息,消息体包含具体的消息内容。
1. 定义消息类
```java
public class MyMessage {
private int length;
private String content;
// getter and setter methods
}
```
2. 编写编解码器
```java
public class MyMessageCodec extends MessageToByteEncoder<MyMessage> {
@Override
protected void encode(ChannelHandlerContext ctx, MyMessage msg, ByteBuf out) throws Exception {
byte[] contentBytes = msg.getContent().getBytes(CharsetUtil.UTF_8);
out.writeInt(contentBytes.length);
out.writeBytes(contentBytes);
}
}
public class MyMessageDecoder extends ByteToMessageDecoder {
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List<Object> out) throws Exception {
if (in.readableBytes() < 4) {
return;
}
in.markReaderIndex();
int length = in.readInt();
if (in.readableBytes() < length) {
in.resetReaderIndex();
return;
}
byte[] contentBytes = new byte[length];
in.readBytes(contentBytes);
String content = new String(contentBytes, CharsetUtil.UTF_8);
MyMessage message = new MyMessage();
message.setLength(length);
message.setContent(content);
out.add(message);
}
}
```
3. 编写服务端和客户端代码
服务端代码:
```java
public class MyServer {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyMessageDecoder());
pipeline.addLast(new MyServerHandler());
pipeline.addLast(new MyMessageCodec());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(8888).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
public class MyServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
MyMessage message = (MyMessage) msg;
System.out.println("Server receive message: " + message.getContent());
message.setContent("Hello, " + message.getContent() + "!");
ctx.writeAndFlush(message);
}
}
```
客户端代码:
```java
public class MyClient {
public static void main(String[] args) throws Exception {
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(workerGroup)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyMessageCodec());
pipeline.addLast(new MyMessageDecoder());
pipeline.addLast(new MyClientHandler());
}
});
ChannelFuture f = b.connect("localhost", 8888).sync();
for (int i = 0; i < 10; i++) {
MyMessage message = new MyMessage();
message.setContent("world-" + i);
f.channel().writeAndFlush(message);
Thread.sleep(1000);
}
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
}
}
}
public class MyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
MyMessage message = (MyMessage) msg;
System.out.println("Client receive message: " + message.getContent());
}
}
```
以上代码演示了如何使用Netty实现自定义协议,其中MyMessageCodec和MyMessageDecoder负责编解码,MyServer和MyServerHandler负责服务端逻辑,MyClient和MyClientHandler负责客户端逻辑。在本示例中,自定义协议包含消息头和消息体,消息头包含消息长度信息,消息体包含具体的消息内容。
netty对接modbus协议
Netty是一个高性能的网络通信框架,可以用于将Modbus协议与网络进行对接。Modbus是一种通信协议,常用于工业领域的设备间通信。下面是一个简单的示例,展示如何使用Netty对接Modbus协议。
首先,你需要引入Netty和Modbus相关的依赖。可以在项目的构建文件(例如pom.xml)中添加以下依赖:
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.63.Final</version>
</dependency>
<dependency>
<groupId>de.gandev</groupId>
<artifactId>modbus-client</artifactId>
<version>2.1.0</version>
</dependency>
```
接下来,你可以创建一个Netty的客户端来连接Modbus设备。以下是一个简单的示例代码:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import de.gandev.modjdatabus.ModbusTcpClient;
import de.gandev.modjdatabus.ModbusTcpClientHandler;
public class ModbusClient {
private final String host;
private final int port;
public ModbusClient(String host, int port) {
this.host = host;
this.port = port;
}
public void run() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 添加Modbus协议处理器
pipeline.addLast(new ModbusTcpClientHandler());
}
});
// 连接Modbus设备
ChannelFuture future = bootstrap.connect(host, port).sync();
Channel channel = future.channel();
// 发送Modbus命令
byte[] command = new byte[]{0x01, 0x03, 0x00, 0x00, 0x00, 0x01, (byte) 0x84, (byte) 0x0A};
channel.writeAndFlush(command);
// 等待连接关闭
future.channel().closeFuture().sync();
} finally {
// 关闭连接
group.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
String host = "192.168.0.1";
int port = 502;
ModbusClient client = new ModbusClient(host, port);
client.run();
}
}
```
在上述示例中,我们首先创建了一个`ModbusTcpClientHandler`,它是一个Netty的ChannelHandler,用于处理Modbus协议的请求和响应。然后,我们使用Netty的`Bootstrap`来设置客户端的配置和处理器。最后,我们连接Modbus设备并发送Modbus命令。
请注意,以上只是一个简单的示例,实际的Modbus通信可能涉及更多的细节和配置。你可以根据具体的需求进行修改和扩展。希望对你有所帮助!
阅读全文
相关推荐
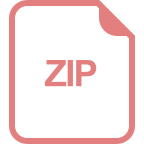
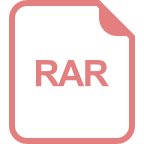
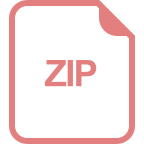













