python re 字符匹配替换
时间: 2023-08-07 16:00:33 浏览: 48
你可以使用Python的re模块来进行字符匹配和替换操作。re模块提供了强大的正则表达式功能,可以用于查找、匹配和替换字符串中的特定模式。
要进行字符匹配替换,你需要使用re.sub()函数。这个函数接受三个参数:匹配的模式、替换的字符串和要搜索的字符串。它会将匹配到的模式替换为指定的字符串。
下面是一个使用re.sub()进行字符匹配替换的示例:
```python
import re
string = "Hello, World! Hello, Python!"
pattern = r"Hello"
replacement = "Hi"
new_string = re.sub(pattern, replacement, string)
print(new_string)
```
这段代码将会输出:"Hi, World! Hi, Python!"。其中,r"Hello"是我们要匹配的模式,"Hi"是替换的字符串,而"Hello, World! Hello, Python!"是要搜索的字符串。
需要注意的是,re.sub()函数会将所有匹配到的模式替换为指定的字符串。如果你只想替换第一个匹配到的模式,可以使用re.sub()的count参数,将其设置为1。
希望以上信息能对你有所帮助!如果你还有其他问题,请随时提问。
相关问题
python替换字符串re
回答: 在Python中,可以使用re.sub方法来替换字符串。re.sub方法通过正则表达式匹配字符串中的某个模式,并将其替换为指定的字符串。re.sub方法的语法如下:re.sub(pattern, repl, string)。其中,pattern是要匹配的模式,repl是替换的字符串,string是要进行替换的原始字符串。使用re.sub方法可以在字符串中找到匹配的模式,并将其替换为指定的字符串。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Python字符串替换的3种方法](https://blog.csdn.net/qdPython/article/details/130137565)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
python的字符串替换
使用Python进行字符串替换有多种方法,以下是其中几种常用的方式:
1. 使用replace()函数:该函数可以用来替换字符串中的指定子串。它接受两个参数,第一个参数是要被替换的子串,第二个参数是用来替换的新字符串。示例代码如下:
```python
string = "Hello, World!"
new_string = string.replace("World", "Python")
print(new_string)
```
输出结果为:"Hello, Python!"
2. 使用re.sub()函数:该函数是正则表达式模块re中的一个方法,可以通过正则表达式来匹配并替换字符串中的内容。示例代码如下:
```python
import re
string = "Hello, World!"
new_string = re.sub("World", "Python", string)
print(new_string)
```
输出结果为:"Hello, Python!"
3. 使用字符串的切片和拼接:可以通过切片的方式获取到需要替换的部分字符串,然后再与新字符串进行拼接。示例代码如下:
```python
string = "Hello, World!"
new_string = string[:7] + "Python" + string[12:]
print(new_string)
```
输出结果为:"Hello, Python!"
以上是几种常用的字符串替换方法,你可以根据具体的需求选择适合你的方法。
相关推荐
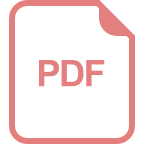
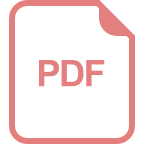
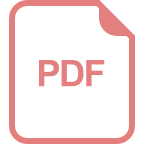












