java编写代码实现+、-、*、\及括号的应用;例如输入实现(a+b)*c-d/2
时间: 2024-09-19 21:06:47 浏览: 57
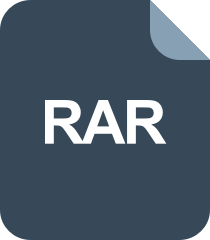
Java编写的计算器图形界面实现加减乘除带括号运算
在Java中,你可以使用表达式解析库如JEP (Java Expression Parser) 或者简单的正则表达式配合字符串处理,来实现类似数学表达式的计算。下面是一个简化的例子,我们将使用`eval`函数来计算给定的字符串:
```java
import java.util.Stack;
import java.lang.reflect.Method;
public class MathExpressionEvaluator {
public static double evaluate(String expression) {
try {
// 将运算符优先级存储在一个栈里
Stack<String> operators = new Stack<>();
String[] tokens = expression.split("(\\+|\\-|\\*|\\/)");
for (String token : tokens) {
if (!token.isEmpty()) { // 检查是否为数字
double num = Double.parseDouble(token);
while (!operators.isEmpty() && hasHigherPrecedence(operators.peek(), token)) {
num = applyOperator(num, operators.pop());
}
operators.push(num + "");
} else if (token.equals("(")) {
operators.push(token);
} else if (token.equals(")")) {
while (!operators.peek().equals("(")) {
num = applyOperator(Double.parseDouble(operators.pop()), Double.parseDouble(operators.pop()));
}
operators.pop(); // 移除左括号
}
}
// 应用剩余的运算符
while (!operators.isEmpty()) {
num = applyOperator(Double.parseDouble(operators.pop()), Double.parseDouble(operators.pop()));
}
return num;
} catch (Exception e) {
throw new RuntimeException("Invalid expression", e);
}
}
private static boolean hasHigherPrecedence(String op1, String op2) {
// 定义运算符的优先级,这里简化为'/'>'*'>'+'>'-'
switch (op1) {
case "*":
case "/":
return op2.equals("+") || op2.equals("-");
default:
return false;
}
}
private static double applyOperator(double b, double a) {
Method m = null;
try {
switch (operators.peek()) {
case "+":
m = Math.class.getMethod("add", a.getClass(), b.getClass());
break;
case "-":
m = Math.class.getMethod("subtract", a.getClass(), b.getClass());
break;
case "*":
m = Math.class.getMethod("multiply", a.getClass(), b.getClass());
break;
case "/":
m = Math.class.getMethod("divide", a.getClass(), b.getClass());
break;
}
} catch (NoSuchMethodException e) {
throw new RuntimeException("Invalid operation");
}
try {
return (Double) m.invoke(null, a, b);
} catch (IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException("Error in applying operator", e);
}
}
public static void main(String[] args) {
String expression = "(a+b)*c-d/2";
System.out.println(evaluate(expression));
}
}
```
请注意,这个例子非常基础,实际应用中可能会涉及到更复杂的错误检查和边界条件处理。此外,如果你不想依赖反射,可以考虑使用第三方库,如JEP或者使用正则表达式来解析表达式。
阅读全文
相关推荐
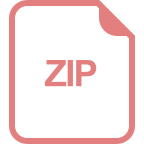
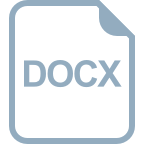





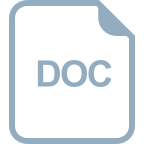
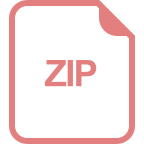
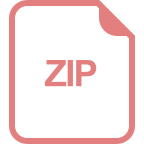
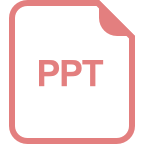
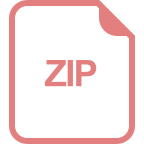
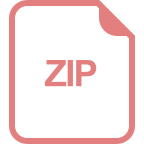
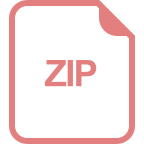
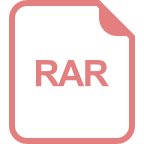
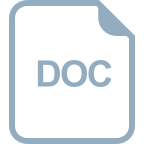

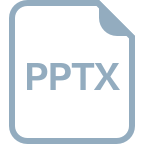