1. 编写Java程序,定义复数类。 (1)复数具有实部、虚部属性; (2)复数类具有构造方法(参数为复数的实部与虚部); (3)复数类具有复数的加、减以及显示复数对象等功能.
时间: 2024-10-18 18:08:34 浏览: 96
为了编写一个简单的Java程序定义复数类(ComplexNumber),我们可以按照以下步骤进行:
```java
public class ComplexNumber {
// 定义复数的实部和虚部属性
private double real;
private double imaginary;
// 构造函数,接受实部和虚部作为参数
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 加法方法
public ComplexNumber add(ComplexNumber other) {
return new ComplexNumber(this.real + other.real, this.imaginary + other.imaginary);
}
// 减法方法
public ComplexNumber subtract(ComplexNumber other) {
return new ComplexNumber(this.real - other.real, this.imaginary - other.imaginary);
}
// 显示复数的方法(通常会重写toString()方法)
@Override
public String toString() {
if (imaginary >= 0) {
return String.format("%.2f + %.2fi", real, imaginary);
} else {
return String.format("%.2f - %.2fi", real, Math.abs(imaginary));
}
}
}
```
在这个`ComplexNumber`类中,我们定义了实部和虚部作为私有变量,并提供了相应的构造方法、加法和减法方法。`toString()`方法用于将复数表示为标准形式如"3.5 + 4.0i"或"3.5 - 4.0i"。
阅读全文
相关推荐
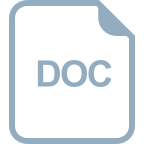
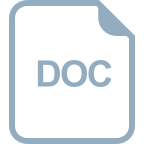
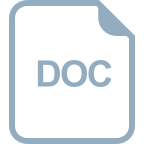
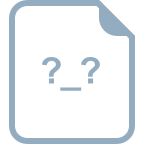
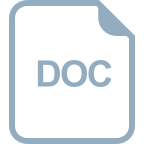
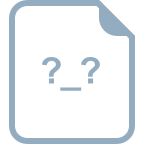
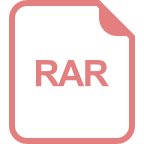
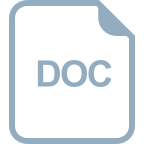
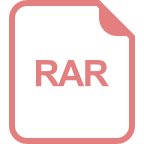









